the problem is, when a trade hits tp and it calculates the (TT) value for partial closing of the opposite position, instead of doing it once, it does it multiple of time until the loss position will be closed completely!!!!
There is a lot of info here so I will focus on the problem statement (apologies if I have missed some detail)
If you set a breakpoint in OnTradeTransaction() I think you will find it is called many times when closing a ticket - you need some way of tracking if you have hedged it already.
There could be a better/standard way, but in my ignorance I have written mechanisms to do this.
For example, you could capture the posID from request.position and add it to a global list and check whether it has been hedged before attempting a new hedge - of course if your EA restarts you would need a way of reconstructing that list - maybe writing to a file could be a simple way to preserve it.
I would also suggest you read this section of the help which may give you some ideas on how you want to approach it
https://www.mql5.com/en/docs/event_handlers/ontradetransaction
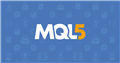
- www.mql5.com
There is a lot of info here so I will focus on the problem statement (apologies if I have missed some detail)
If you set a breakpoint in OnTradeTransaction() I think you will find it is called many times when closing a ticket - you need some way of tracking if you have hedged it already.
There could be a better/standard way, but in my ignorance I have written mechanisms to do this.
For example, you could capture the posID from request.position and add it to a global list and check whether it has been hedged before attempting a new hedge - of course if your EA restarts you would need a way of reconstructing that list - maybe writing to a file could be a simple way to preserve it.
I would also suggest you read this section of the help which may give you some ideas on how you want to approach it
https://www.mql5.com/en/docs/event_handlers/ontradetransaction
Thanks so much for replying.
i tried to do that with a bool variable, and i didn't get what i expected. i will use your idea and check that with position ID, and will share the result here.
thanks again for your time.
hi R4tna
i couldn't find any way to do that with position ID filter and OnTransaction Function, so i decided to write the code differently with OnTick function and i made it work, however that was not the logical solution for that, i continue researching to find the right solution and share it here for others who may have same issue.
thanks anyway
hi R4tna
i couldn't find any way to do that with position ID filter and OnTransaction Function, so i decided to write the code differently with OnTick function and i made it work, however that was not the logical solution for that, i continue researching to find the right solution and share it here for others who may have same issue.
thanks anyway
sure - if that suits you better then it may be the better way
Did you try creating a global variable/array to store the posID when hedging from OnTradeTransaction()?
sure - if that suits you better then it may be the better way
Did you try creating a global variable/array to store the posID when hedging from OnTradeTransaction()?
yes, i defined a global array to store the position ID of the opposite position which was supposed to be closed or partially closed, and at the end of that function i added the position ID to the array, then every time before calling the function, i checked the position id with the array data, but that didn't work for me or perhaps i wrote it wrongly !!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Dear developers, i am trying to write a hedging expert advisor and i have some strange issues with making it work properly,
first let me explain the whole idea and then mention the issue:
suppose LotMax = 1.0 (input box variable)
it is petty much a bot that when it hits its TP it applies some of that profit to its losing positions. the EA opens a random buy or sell position with some simple logics at first, let's imagine it is a buy(lot=LotMax at first run), then it place a sellstop(same lot, =LotMax) 20pips below the buy entry immediately, all positions have no sl and tp is 40 pips for example, if the buy hits tp and sell stop is not triggered it deletes the sell stop and does the previous step again.
But if the sellstop is filled, when buy hits tp, it read the last tp profit (for example 1.0 lot + 40pips == +400$) and decide to use some of it for example +300$ (TT value in my code) for closing the furthest opposite running trade which is in loss, here the 1.0 lot sell is in -60pips loss (about -600$), so if the trade loss is less than TT value it closes it all, if not like here, it does partial exit, so the EA close about half size of sell trade in loss and makes it Sell (0.5 lot)
in this point because the EA has a sell trade, at the closure point of previous trade, it opens a sell (lot= LotMax- summation of sell basket so lot = 1.0 - 0.5 = 0.5) and hedge it with a buy stop 20 pips above with lot size = lotMax- summation of buy basket lots= 1.0 - 0 = 1.0
and so on...
the problem is, when a trade hits tp and it calculates the (TT) value for partial closing of the opposite position, instead of doing it once, it does it multiple of time until the loss position will be closed completely!!!!
i have used OnTradeTransaction function to call close/Partial close function, and i have written the code in many different states, but the issue remains the same, i will attach my code and waiting for your help.
( i deleted some part of the code like position counter and ... in order to not exceed tha max characters for posting here.)
Thanks in advance.