Your topic has been moved to the section: Expert Advisors and Automated Trading
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Hi @ Okeyo Giose
I'm not a professional, but I'll try to help you.
When you have a buy signal, I have the impression that you only buy, but you don't close your short positions if there are any, of course.
For example, you could create a simple function to close shorts, and call it up when you have a long condition.
//+------------------------------------------------------------------+ //| My FonctionS | //+------------------------------------------------------------------+ //--- // Clsoe All Short Position void Close_All_Short_Positions(){ for (int i = PositionsTotal()-1 ; i >= 0 ; i--){ ulong ticket = PositionGetTicket(i) ; long type; if ( type == POSITION_TYPE_BUY) { continue; } trade.PositionClose(ticket); } }
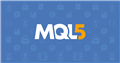
so peroblem solved right ?

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi guys, beginner here new to making EA's. Time to put my pride aside and ask for help, I cant figure this one out.
I want to close any opened buy positions when a sell condition is met, and close any opened sell positions when a buy condition is met. Here's the code! And much appreciated in advance!