Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
I sincerely hope this is not ChatGPT (or other A.I.) generated code. Because if it is, then please, don't request help with it.
It generates horrible code, mixing MQL4 and MQL5. Please use the Freelance section for such requests — https://www.mql5.com/en/job
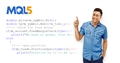
- 2023.11.21
- www.mql5.com
I gave you clear instructions, yet you posted a new post with again improperly formatted code, instead of editing your original post and doing it properly.
That is because your program is incomplete. Where is the closing brace "}" for the OnTick() function?
int BuyOrder = OrderSend(NULL, OP_BUY, 0.08, Ask, 1, Ask - 0.5, Ask + 2.5, NULL, 123456, 0, clrGreen); Print("Buy Order Took Place");
-
Be careful with NULL.
- On MT4, you can use NULL in place of _Symbol only in those calls that the documentation specially says you can. iHigh does, iCustom does, MarketInfo does not, OrderSend does not.
- Don't use NULL (except for pointers where you explicitly check for it.) Use _Symbol and _Period, that is minimalist as possible and more efficient.
- Zero is the same as PERIOD_CURRENT which means _Period. Don't hard code numbers.
- MT4: No need for a function call with iHigh(NULL,0,s) just use the predefined arrays, i.e. High[].
MT5: create them. - Cloud Protector Bug? - MQL4 programming forum (2020)
-
Check your return codes, and report your errors (including market prices and your variables). Don't look at GLE/LE unless you have an error. Don't just silence the compiler (MT5 / MT4+strict), it is trying to help you.
What are Function return values ? How do I use them ? - MQL4 programming forum (2012)
Common Errors in MQL4 Programs and How to Avoid Them - MQL4 Articles (2014)You buy at the Ask and sell at the Bid. Pending Buy Stop orders become market orders when hit by the Ask. -
You buy at the Ask and sell at the Bid. Pending Buy Stop orders become market orders when hit by the Ask.
-
Your buy order's TP/SL (or Sell Stop's/Sell Limit's entry) are triggered when the Bid / OrderClosePrice reaches it. Using Ask±n, makes your SL shorter and your TP longer, by the spread. Don't you want the specified amount used in either direction?
-
Your sell order's TP/SL (or Buy Stop's/Buy Limit's entry) will be triggered when the Ask / OrderClosePrice reaches it. To trigger close at a specific Bid price, add the average spread.
MODE_SPREAD (Paul) - MQL4 programming forum - Page 3 #25 -
The charts show Bid prices only. Turn on the Ask line to see how big the spread is (Tools → Options (control+O) → charts → Show ask line.)
Most brokers with variable spreads widen considerably at end of day (5 PM ET) ± 30 minutes.
My GBPJPY shows average spread = 26 points, average maximum spread = 134.
My EURCHF shows average spread = 18 points, average maximum spread = 106.
(your broker will be similar).
Is it reasonable to have such a huge spreads (20 PIP spreads) in EURCHF? - General - MQL5 programming forum (2022)
-
bool isCondition1 = PRICE_CLOSE >= Lips_0 && Lips_0 > Teeth_0 && Teeth_0 > Jaws_0 && Lips_0 > Jaws_0 && PRICE_CLOSE >= Lips_1 && Lips_1 > Teeth_1 && Teeth_1 > Jaws_1 && Lips_1 > Jaws_1 && PRICE_CLOSE >= Lips_2 && Lips_2 > Teeth_2 && Teeth_2 > Jaws_2 && Lips_2 > Jaws_2;PRICE_CLOSE is the constant zero. Get the actual price.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I keep getting unexpected end to program and unbalanced parenthesis, anyone see the issue? I'm not sure where the snag is, thanks for any help.