- Closing position in an array
- Array out of range. But why?
- Array out of range error
You have declared an dynamic array, which will be empty (size = 0).
double profitarray[][2];
Yet you immediately begin to try assigning values to its elements ...
profitarray[i][0]=m_position.Ticket(); profitarray[i][1]=m_position.Profit();
So obviously it will give an array out of range error.
You will need to first set the size of the dynamic array accordingly, before populating it ...
Sets the new size in the first dimension of the array
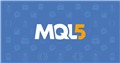
- www.mql5.com
Many thanks Fernando, I have implemented this and cleared the array resize issue, now I am getting the following error during the running of the EA:
VirtualAlloc failed in large allocator size=2684354592
I am fairly new to MQL/C++ and haven't quite got to grips with the memory allocation side of things, is this due to array sizes or variable sizes?
The memory allocation problem has been solved, however the EA now just freezes when this function is called, does anyone know what could be causing this?
I get the following warning on compilation;
implicit conversion from 'number' to 'string'
I think this issue has something to do with the PositionClose function but I do not know what or how to fix this
Then show your new code and the the log output and identify the affected line numbers.
We cannot read your mind nor see your computer.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use