Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
There may be a common bug in MQL5 indicator functions or I'm missing something. The problem arises when I try to apply any indicator on any custom indicator. For example, in MQL4, you calculate the moving average of any indicator by first filling an array with the indicator and then calling the function iMAOnArray(). In MQL5, there's no such function iMAOnArray(), but you put the handle of the indicator as the last parameter of the iMA function. This works well for standard indicators, but not for custom indicators.
Note the two lines with "custom_indicator_handle = " in the code below. First, I try to assign it to a Moving Average and then I use it as the last parameter in another iMA function. This calculates the moving average of a moving average which works fine and the line is displayed on the chart.
Then I commented this line and I assigned it to a custom indicator instead. After that, no line is displayed on the chart and the indicator value is always "inf" in the Data Window.
Custom indicator code:
For demonstration, I created a basic custom indicator that just copies the Moving Average indicator, so the code above should calculate "moving average of a moving average", but here the moving average is a custom indicator.
The custom indicator is correctly displayed as a line, so the buffer is filled and I see no reason why it can't be used as a handle of the iMA function.
I attach both codes as files.
There are no common bugs in the MQL5 indicator, that happened is because you don't know how to implement iMAOnArray() in MQL5.
To implement the custom indicator that you created, use include file:
#include <MovingAverages.mqh>
How to use it, please learn lots of examples in CodeBase, or the easiest way is to see it in the MACD indicator code.
PlotIndexSetInteger(0, PLOT_DRAW_BEGIN, 15);
I have another, somewhat related problem here:
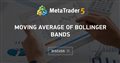
- 2023.10.08
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
There may be a common bug in MQL5 indicator functions or I'm missing something. The problem arises when I try to apply any indicator on any custom indicator. For example, in MQL4, you calculate the moving average of any indicator by first filling an array with the indicator and then calling the function iMAOnArray(). In MQL5, there's no such function iMAOnArray(), but you put the handle of the indicator as the last parameter of the iMA function. This works well for standard indicators, but not for custom indicators.
Note the two lines with "custom_indicator_handle = " in the code below. First, I try to assign it to a Moving Average and then I use it as the last parameter in another iMA function. This calculates the moving average of a moving average which works fine and the line is displayed on the chart.
Then I commented this line and I assigned it to a custom indicator instead. After that, no line is displayed on the chart and the indicator value is always "inf" in the Data Window.
Custom indicator code:
For demonstration, I created a basic custom indicator that just copies the Moving Average indicator, so the code above should calculate "moving average of a moving average", but here the moving average is a custom indicator.
The custom indicator is correctly displayed as a line, so the buffer is filled and I see no reason why it can't be used as a handle of the iMA function.
I attach both codes as files.