If your program (EA or indicator) doesn't do what it should use the debugger (in case of an indicator make sure it is not running on a different chart of the terminal):
Code debugging: https://www.metatrader5.com/en/metaeditor/help/development/debug
Error Handling and Logging in MQL5: https://www.mql5.com/en/articles/2041
Tracing, Debugging and Structural Analysis of Source Code, scroll down to: "Launching and Debuggin": https://www.mql5.com/en/articles/272
- www.metatrader5.com
If your program (EA or indicator) doesn't do what it should use the debugger (in case of an indicator make sure it is not running on a different chart of the terminal):
Code debugging: https://www.metatrader5.com/en/metaeditor/help/development/debug
Error Handling and Logging in MQL5: https://www.mql5.com/en/articles/2041
Tracing, Debugging and Structural Analysis of Source Code, scroll down to: "Launching and Debuggin": https://www.mql5.com/en/articles/272
Hey Carl,
Thanks for the reply.
I already used the debugger, as mentioned in my initial message. The indicator buffer is not returned on initialization and on timeframe change (re-initialization) --> 4806 Requested data not found.
However, leaving it there for the next candle it is being copied with no problem. This conclusion was made from buffer reading and behavior on charts.
Both cases above are using the same exact function. I am wondering if it is a kind of limitation where the indicator buffer is not loaded correctly when the timeframe used is different than the chart timeframe. As I am using standard MT5 calls to initiate the indicator and call its buffers.
I adjusted this part of my code just to throw errors in the journal, just to make my analysis more readable - check the gif attached simulating the scenarios.
if(result == 1 ) {fractal = mBuffer[0]; Print(i,":",fractal);} else Print(i,":",GetLastError());
What I suspect is there is something in MQL5 libraries, not allowing the buffer to correctly being initialized .
Risk a look in other indicators in the code base.
Nothing happens in OnCalculate() until the 1st new bar appears - where is the calculation of the history of the chart?
Check the orientation of all your buffers (oldest and most recent values). See the doc. of CopyBuffer().
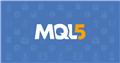
- www.mql5.com
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi,
I am building an indicator to display several visual objects on the graph. I have a problem getting the high and low defined by ZigZag from a higher timeframe.
My indicator will not use a buffer to save data, I want it to plot objects on the chart and will do the object plotting on initialization and on each new candle only.
I know it is not a usual thing for indicators, but please bear with me to explain the issue I am facing.
When loading the indicator on the screen, nothing happens, and when debugging, I have all the buffers returned as zero. Same issue when changing timeframes.
However leaving it on the chart for one new candle, everything seems to work right and I have the object plotted.
Anything I am doing wrong? The same function is called in both initialization and new bar, so it is kind of weird. Would it be a problem handling buffers on initialization?
Thanks for the help