the Editor has a profiler to find where a program looses time and where it can be improved:
Beside that learn to use the debugger: https://www.metatrader5.com/en/metaeditor/help/development/debug
- www.metatrader5.com
- What is numBufferCopy? Don't post code that will not even compile.
- Perhaps it is your indicator. Code it properly so it only recomputes bar zero (after the initial run).
How to do your lookbacks correctly. (2016)
3 Methods of Indicators Acceleration by the Example of the Linear Regression - MQL5 Articles. (2011)
Or, reduce Tools → Options (control+O) → Charts → Max bars in chart to something reasonable (like 1K.)
the Editor has a profiler to find where a program looses time and where it can be improved:
Beside that learn to use the debugger: https://www.metatrader5.com/en/metaeditor/help/development/debug
I try the profile and it seems to be spending 91% of the time on the first CopyBuffer() call.
I tried putting a breakpoint either side of the first CopyBuffer() call but wasn't sure what to look for - it looks as if it is copying the buffer as intended and it is not particularly slow (it clocked 0.1 sec in the run just now)
- What is numBufferCopy? Don't post code that will not even compile.
- Perhaps it is your indicator. Code it properly so it only recomputes bar zero (after the initial run).
How to do your lookbacks correctly. (2016)
3 Methods of Indicators Acceleration by the Example of the Linear Regression - MQL5 Articles. (2011)
Or, reduce Tools → Options (control+O) → Charts → Max bars in chart to something reasonable (like 1K.)
My apologies - I missed out a bunch of variable declarations at the top of the EA and have included them back now.
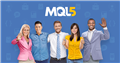
- www.mql5.com
" I try the profile and it seems to be spending 91% of the time on the first CopyBuffer() call."
Then it seems that the problem is the indicator!
Maybe you should read:
Tips from a professional programmer (Part I): Code storing, debugging and compiling. Working with projects and logs
https://www.mql5.com/en/articles/9266
Tips from a professional programmer (Part II): Storing and exchanging parameters between an Expert Advisor, scripts and external programs
https://www.mql5.com/en/articles/9327
Tips from a professional programmer (Part III): Logging. Connecting to the Seq log collection and analysis system
https://www.mql5.com/en/articles/10475
Also study the indicators on your pc in .....\MQL5\Indicators\Examples\ ...
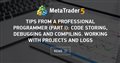
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi, I am relatively new to MT5 and having my first attempt to write an experimental EA after reading the tutorials and examples here.
I am trying to initialize multiple instance of a customed indicator with different trend period using iCustom(), and trying to process the indicator value using BufferCopy() at each tick within OnTick().
It seems to work with 1 instance of the indicator, but as soon as I added addition instances of the indicators (with different parameters), I noticed I start to miss ticks and in particulare see far less ticks (like 1 tick per half minute vs the normal 50+ tick per minute I see at on seperate screen at the same moment) being processed in the debug log even with just 2 indicators and gradually got worst as I add more.
Below is the code for my test EA
I know it is by design that MT5 will miss ticks when busy processing OnTick(), but with the OnTick() processing took approx. 0.2sec for processing 6 iCustom indicators, it seem excessively that it is only picking up ticks every 30 second-ish.
Is there something I am doing wrong or I should be careful when processing multiple iCustom indicators to minimized tick being missed?
Many thanks in advance,
Anthony