Here are the tools to find the problems in your code:
Code debugging: https://www.metatrader5.com/en/metaeditor/help/development/debug
Error Handling and Logging in MQL5: https://www.mql5.com/en/articles/2041
Tracing, Debugging and Structural Analysis of Source Code, scroll down to: "Launching and Debuggin": https://www.mql5.com/en/articles/272
- www.metatrader5.com
Here are the tools to find the problems in your code:
Code debugging: https://www.metatrader5.com/en/metaeditor/help/development/debug
Error Handling and Logging in MQL5: https://www.mql5.com/en/articles/2041
Tracing, Debugging and Structural Analysis of Source Code, scroll down to: "Launching and Debuggin": https://www.mql5.com/en/articles/272
Thanks, very helpfull
I've noticed its the variable close ... when it reaches the first if, its value changes to 1, and don't know why or how to fix this. Can you tell me whats happening?
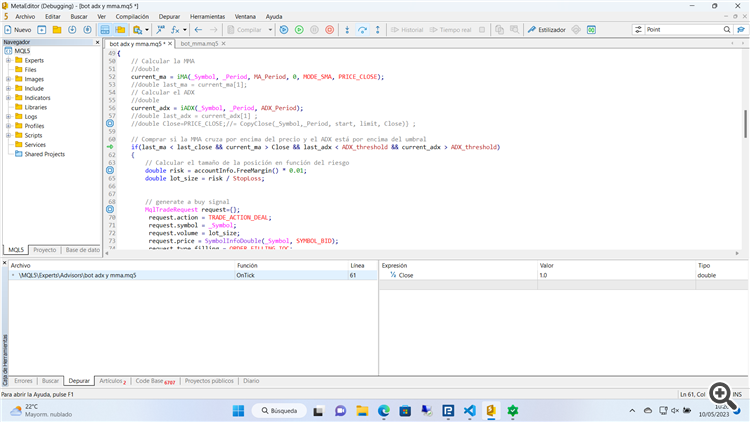
if (even though commented) close = PRICE_CLOSE it demonstrates you have no idea about MQL5
As I said place the cursor on that colored word PRICE_CLOSE (where it's not commented) and press F1.
You should learn to code:
All: https://www.mql5.com/en/forum/11134
Quickstart for newbies: https://www.mql5.com/de/articles/496
and: https://www.mql5.com/de/articles/100
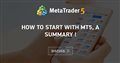
- 2013.03.15
- www.mql5.com
void OnTick() { // Calcular la MMA //double current_ma = iMA(_Symbol, _Period, MA_Period, 0, MODE_SMA, PRICE_CLOSE); //double last_ma = current_ma[1]; // Calcular el ADX //double current_adx = iADX(_Symbol, _Period, ADX_Period);
Those functions do not return a double.
Perhaps you should read the manual, especially the examples.
How To Ask Questions The Smart Way. (2004)
How To Interpret Answers.
RTFM and STFW: How To Tell You've Seriously Screwed Up.
They all (including iCustom) return a handle (an int). You get that in OnInit. In OnTick/OnCalculate (after the indicator has updated its buffers), you use the handle, shift and count to get the data.
Technical Indicators - Reference on algorithmic/automated trading language for MetaTrader 5
Timeseries and Indicators Access / CopyBuffer - Reference on algorithmic/automated trading language for MetaTrader 5
How to start with MQL5 - General - MQL5 programming forum - Page 3 #22 (2020)
How to start with MQL5 - MetaTrader 5 - General - MQL5 programming forum - Page 7 #61 (2020)
MQL5 for Newbies: Guide to Using Technical Indicators in Expert Advisors - MQL5 Articles (2010)
How to call indicators in MQL5 - MQL5 Articles (2010)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
no errors, but no trades are done while testing ... why?