This generates the following Output in the Terminal : 2023.04.13 09:51:50.356 mt5_chart_marker (EURUSD,M15) Header: 猢浡汰彥楴敭栬彴楴敭栬彴灯湥栬彴楨桧栬彴潬ⱷ瑨损潬敳栬彴潢汬湩敧彲業Ɽ瑨佟䱈彃慭ⱸ瑨佟䱈彃業Ɱ瑨江煩瑟灹ⱥ瑬瑟浩ⱥ瑬潟数Ɱ瑬桟杩ⱨ瑬江睯氬彴汣獯ⱥ瑬扟汯楬杮牥浟摩氬彴䡏䍌浟硡氬彴䡏䍌浟湩氬彴慶楬彤慲杮ⱥ瑬瑟灡牟湡敧∬搢獩㵴ⰰ牰硯ㄽ∢琬灡数彤祢猬ⱬ湥牴ⱹ灴搬物ഢㄊ㤹⸹ㄮ‵〲㐺㨵〰ㄬ㤹⸹ㄮ‵㈱〺㨰〰ㄬ㘮㠵ⰳ⸱㘶㘲ㄬ㘮㠴ⰵ⸱㔶ㄵㄬ㘮㘴㌴ㄬ㘮㈶ⰶ⸱㐶㔸ⰱ㤱㤹〮⸱㔱ㄠ㨴〰〺ⰰ⸱㔶㔶ㄬ㘮㠵ⰶ⸱㐶㔸ㄬ㘮〵ⰲ⸱㔶㈸㔴ㄬ㘮㠵ⰶ⸱㐶㔸ㄬ㘮㠴ⰵ⸱㐶㘹㘰㘶ⰷⰰ㤱㤹〮⸱㔱㈠㨰㔱〺ⰰ⸱㐶㤸㈰ㄬ㘮㤴㜳ㄬ㘮㘵ㄲⰵ潬杮㤱㤹〮⸱㤱〠㨰〳〺ⰰ㤱㤹〮⸱㠱ㄠ㨶〰〺ⰰ⸱㔶㠳ㄬ㘮㐵ⰸ⸱㔶ㄱㄬ㘮㌵ⰴ⸱㔶㤲ⰳ⸱㔶㠴ㄬ㘮ㄵⰱㄭㄬ㤹⸹ㄮ‸㜱ㄺ㨵〰ㄬ㘮㈵ⰴ⸱㔶㈳ㄬ㘮ㄵⰱ⸱㔶㤱ㄬ㘮㐵㈰ⰵ⸱㔶㈳ㄬ㘮ㄵⰱ⸱㔶ㄱㄬ㘮ㄵ㈴〬ㄬ㤹⸹ㄮ‹〰ㄺ㨵〰ㄬ㘮ㄵ㌱ⰴ⸱㔶㈱ⰹ⸱㔶㘴㔵氬湯൧ㄊ㤹⸹ㄮ‹㘰㌺㨰〰ㄬ㤹⸹ㄮ‸㠰〺㨰〰ㄬ㘮㌵ⰲ⸱㔶㐵ㄬ㘮㤴ⰹ⸱㔶㘴ㄬ㘮ㄵⰸ⸱㔶㐵ㄬ㘮㤴ⰹㄭㄬ㤹⸹ㄮ‸㤰㐺㨵〰ㄬ㘮㌵ⰳ⸱㔶㔳ㄬ㘮㤴ⰹ⸱㔶㈱ㄬ㘮㈵㐳ㄬ㘮㌵ⰵ⸱㐶㤹ㄬ㘮㤴ⰹ⸱㔶㐰ⰶⰱ㤱㤹〮⸱㤱〠㨵〳〺ⰰ⸱㔶㐵ㄬ㘮㤴㤷ㄬ㘮㘴ㄹⰵ桳牯൴ㄊ㤹⸹ㄮ‹㌱㌺㨰〰ㄬ㤹⸹ㄮ‹㠰〺㨰〰ㄬ㘮
Which should be: "sample_time,ht_time,ht_open,ht_high,ht_low,ht_close,ht_bollinger_mid,ht_OHLC_max,ht_OHLC_min,ht_liq_type,lt_time,lt_open,lt_high,lt_low,lt_close,lt_bollinger_mid,lt_OHLC_max,lt_OHLC_min,lt_valid_range,lt_tap_range,""dist=0,prox=1"",tapped_by,sl,entry,tp,dir"
What am I doing wrong?
I use this to read the whole csv file into an array of lines:
int getCsvLines( const string fName, string &l[], int flag=0, ushort sepLine='\n') { // alternative to FileOpen uchar Bytes[]; return( FileLoad(fName, Bytes, flag) ? StringSplit(CharArrayToString(Bytes), sepLine, l) : 0); }Hereafter you can split the lines into cells with StringSplit(..)
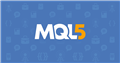
- www.mql5.com
int file_handle = FileOpen(filename, FILE_READ | FILE_CSV, ',');
- Not specifying whether the file is ANSI or Unicode.
- Your reads already split each field. You can't read the entire line and then split. Simplify your code or change the separator.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
This generates the following Output in the Terminal : 2023.04.13 09:51:50.356 mt5_chart_marker (EURUSD,M15) Header: 猢浡汰彥楴敭栬彴楴敭栬彴灯湥栬彴楨桧栬彴潬ⱷ瑨损潬敳栬彴潢汬湩敧彲業Ɽ瑨佟䱈彃慭ⱸ瑨佟䱈彃業Ɱ瑨江煩瑟灹ⱥ瑬瑟浩ⱥ瑬潟数Ɱ瑬桟杩ⱨ瑬江睯氬彴汣獯ⱥ瑬扟汯楬杮牥浟摩氬彴䡏䍌浟硡氬彴䡏䍌浟湩氬彴慶楬彤慲杮ⱥ瑬瑟灡牟湡敧∬搢獩㵴ⰰ牰硯ㄽ∢琬灡数彤祢猬ⱬ湥牴ⱹ灴搬物ഢㄊ㤹⸹ㄮ‵〲㐺㨵〰ㄬ㤹⸹ㄮ‵㈱〺㨰〰ㄬ㘮㠵ⰳ⸱㘶㘲ㄬ㘮㠴ⰵ⸱㔶ㄵㄬ㘮㘴㌴ㄬ㘮㈶ⰶ⸱㐶㔸ⰱ㤱㤹〮⸱㔱ㄠ㨴〰〺ⰰ⸱㔶㔶ㄬ㘮㠵ⰶ⸱㐶㔸ㄬ㘮〵ⰲ⸱㔶㈸㔴ㄬ㘮㠵ⰶ⸱㐶㔸ㄬ㘮㠴ⰵ⸱㐶㘹㘰㘶ⰷⰰ㤱㤹〮⸱㔱㈠㨰㔱〺ⰰ⸱㐶㤸㈰ㄬ㘮㤴㜳ㄬ㘮㘵ㄲⰵ潬杮㤱㤹〮⸱㤱〠㨰〳〺ⰰ㤱㤹〮⸱㠱ㄠ㨶〰〺ⰰ⸱㔶㠳ㄬ㘮㐵ⰸ⸱㔶ㄱㄬ㘮㌵ⰴ⸱㔶㤲ⰳ⸱㔶㠴ㄬ㘮ㄵⰱㄭㄬ㤹⸹ㄮ‸㜱ㄺ㨵〰ㄬ㘮㈵ⰴ⸱㔶㈳ㄬ㘮ㄵⰱ⸱㔶㤱ㄬ㘮㐵㈰ⰵ⸱㔶㈳ㄬ㘮ㄵⰱ⸱㔶ㄱㄬ㘮ㄵ㈴〬ㄬ㤹⸹ㄮ‹〰ㄺ㨵〰ㄬ㘮ㄵ㌱ⰴ⸱㔶㈱ⰹ⸱㔶㘴㔵氬湯൧ㄊ㤹⸹ㄮ‹㘰㌺㨰〰ㄬ㤹⸹ㄮ‸㠰〺㨰〰ㄬ㘮㌵ⰲ⸱㔶㐵ㄬ㘮㤴ⰹ⸱㔶㘴ㄬ㘮ㄵⰸ⸱㔶㐵ㄬ㘮㤴ⰹㄭㄬ㤹⸹ㄮ‸㤰㐺㨵〰ㄬ㘮㌵ⰳ⸱㔶㔳ㄬ㘮㤴ⰹ⸱㔶㈱ㄬ㘮㈵㐳ㄬ㘮㌵ⰵ⸱㐶㤹ㄬ㘮㤴ⰹ⸱㔶㐰ⰶⰱ㤱㤹〮⸱㤱〠㨵〳〺ⰰ⸱㔶㐵ㄬ㘮㤴㤷ㄬ㘮㘴ㄹⰵ桳牯൴ㄊ㤹⸹ㄮ‹㌱㌺㨰〰ㄬ㤹⸹ㄮ‹㠰〺㨰〰ㄬ㘮
Which should be: "sample_time,ht_time,ht_open,ht_high,ht_low,ht_close,ht_bollinger_mid,ht_OHLC_max,ht_OHLC_min,ht_liq_type,lt_time,lt_open,lt_high,lt_low,lt_close,lt_bollinger_mid,lt_OHLC_max,lt_OHLC_min,lt_valid_range,lt_tap_range,""dist=0,prox=1"",tapped_by,sl,entry,tp,dir"
What am I doing wrong?