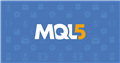
- www.mql5.com
- expert advisor - miscellaneous questions
- Need some help with my simple alert indicator
- Any questions from newcomers on MQL4 and MQL5, help and discussion on algorithms and codes
Please edit your post (don't create a new post) and replace your code properly (with "</>" or Alt-S), or attach the original file directly with the "+ Attach file" button below the text box.
NB! Very important! DO NOT create a new post. EDIT your original post.
This code will not compile! It is full of errors. Is this supposed to be MQL5 or MQL4?
Is this yet another ChatGPT generated code?
If so, then please don't waste our time with this. Hire a proper coder to do the job for you.
This code will not compile! It is full of errors. Is this supposed to be MQL5 or MQL4?
Is this yet another ChatGPT generated code?
If so, then please don't waste our time with this. Hire a proper coder to do the job for you.
NB! Very important! DO NOT create a new post. EDIT your original post.
NB! Very important! DO NOT create a new post. EDIT your original post.
NB! Very important! DO NOT create a new post. EDIT your original post.NB! Very important! DO NOT create a new post. EDIT your original post.
NB! Very important! DO NOT create a new post. EDIT your original post.
NB! Very important! DO NOT create a new post. EDIT your original post.i'm sorryI'm sorry , but i don't undrestad what do you mean , can you be more simplified
It was simple. It couldn't have been clearer.
What part of “edit your original post” was unclear? What part of “DO NOT create a new post” was unclear? Why didn't you do the former, but instead did the latter?
Now, delete your post #4 and fix the original post and use the CODE button (or Alt+S)!
General rules and best pratices of the Forum. - General - MQL5 programming forum (2019)
Messages Editor
// Input parameters input int TableWidth = 200; input int TableHeight = 500; input color TableColor = LightGray; input color HeaderColor = Gray; input color TextColor = Black; input color SelectedPairColor = Red; input color TimeframeColor = Blue; input int FontSize = 10; // Global variables string pairs[] = {"EURUSD", "USDJPY", "GBPUSD", "USDCHF", "AUDUSD", "USDCAD", "NZDUSD", "EURJPY", "EURGBP", "EURCHF", "EURAUD", "EURNZD", "EURCAD", "GBPJPY", "GBPCHF", "GBPAUD", "GBPNZD", "GBPCAD", "CHFJPY", "AUDJPY", "CADJPY", "NZDJPY", "AUDCHF", "AUDCAD", "AUDNZD", "CADCHF", "NZDCHF", "NZDCAD", "XAUUSD"}; int TableX, TableY, CellWidth, CellHeight, CurrentPairIndex, PreviousClosePrice; double PercentChange; color CurrentTimeframeColor; bool IsMovingVerticalLine; // Initialization function int OnInit() { // Calculate table position and cell dimensions TableX = ChartGetInteger(0, CHART_WIDTH_IN_PIXELS) - TableWidth - 5; TableY = 5; CellWidth = TableWidth / 2; CellHeight = TableHeight / 28; // Set chart properties ChartSetInteger(0, CHART_SHIFT, 0); ChartSetInteger(0, CHART_AUTOSCROLL, false); ChartSetInteger(0, CHART_BRING_TO_TOP, true); ChartSetInteger(0, CHART_SHOW_TRADE_LEVELS, false); ChartSetInteger(0, CHART_SHOW_PERIOD_SEP, false); // Create table background ObjectCreate("table_bg", OBJ_RECTANGLE_LABEL, 0, 0, 0); ObjectSet("table_bg", OBJPROP_XDISTANCE, TableX); ObjectSet("table_bg", OBJPROP_YDISTANCE, TableY); ObjectSet("table_bg", OBJPROP_CORNER, 0); ObjectSet("table_bg", OBJPROP_BACK, true); ObjectSet("table_bg", OBJPROP_COLOR, TableColor); ObjectSet("table_bg", OBJPROP_WIDTH, TableWidth); ObjectSet("table_bg", OBJPROP_HEIGHT, TableHeight); // Create table header ObjectCreate("table_header", OBJ_RECTANGLE_LABEL, 0, 0, 0); ObjectSet("table_header", OBJPROP_XDISTANCE, TableX); ObjectSet("table_header", OBJPROP_YDISTANCE, TableY); ObjectSet("table_header", OBJPROP_CORNER, 0); ObjectSet("table_header", OBJPROP_BACK, true); ObjectSet("table_header", OBJPROP_COLOR, HeaderColor); ObjectSet("table_header", OBJPROP_WIDTH, TableWidth); ObjectSet("table_header", OBJPROP_HEIGHT, CellHeight); // Create table cells for (int i = 0; i < 28; i++) { ObjectCreate("table_cell_" + i, OBJ_LABEL, 0, 0, 0); ObjectSet("table_cell_" + i, OBJPROP_CORNER, 0); ObjectSet("table_cell_" + i, OBJPROP_XDISTANCE, TableXDistance + (i % 2) * CellWidth); ObjectSet("table_cell_" + i, OBJPROP_YDISTANCE, TableYDistance + (i / 2) * CellHeight); ObjectSet("table_cell_" + i, OBJPROP_BACK, true); ObjectSet("table_cell_" + i, OBJPROP_COLOR, TextColor); ObjectSet("table_cell_" + i, OBJPROP_SELECTABLE, true); ObjectSet("table_cell_" + i, OBJPROP_SELECTED, false); ObjectSetText("table_cell_" + i, pairs[i], CellWidth - 5, CellHeight, TL_LEFT); ObjectCreate("table_cell_" + i + "_value", OBJ_LABEL, 0, 0, 0); ObjectSet("table_cell_" + i + "_value", OBJPROP_CORNER, 0); ObjectSet("table_cell_" + i + "_value", OBJPROP_XDISTANCE, TableXDistance + CellWidth + (i % 2) * CellWidth); ObjectSet("table_cell_" + i + "_value", OBJPROP_YDISTANCE, TableYDistance + (i / 2) * CellHeight); ObjectSet("table_cell_" + i + "_value", OBJPROP_BACK, true); ObjectSet("table_cell_" + i + "_value", OBJPROP_COLOR, TextColor); ObjectSetText("table_cell_" + i + "_value", DoubleToStr(SymbolInfoDouble(pairs[i], SYMBOL_CLOSE), Digits), CellWidth - 5, CellHeight, TL_RIGHT); // Set table cell properties ObjectSet("table_cell_" + i, OBJPROP_XDISTANCE, TableX); ObjectSet("table_cell_" + i, OBJPROP_YDISTANCE, TableY + CellHeight * (i + 1)); ObjectSet("table_cell_" + i, OBJPROP_CORNER, 0); ObjectSet("table_cell_" + i, OBJPROP_BACK, true); ObjectSet("table_cell_" + i, OBJPROP_COLOR, TextColor); ObjectSet("table_cell_" + i, OBJPROP_FONTSIZE, FontSize); ObjectSetText("table_cell_" + i, pairs[i], CellWidth - 5, CellHeight, TL_RIGHT); ObjectCreate("table_cell_" + i + "value", OBJ_LABEL, 0, 0, 0); ObjectSet("table_cell" + i + "value", OBJPROP_XDISTANCE, TableX + CellWidth); ObjectSet("table_cell" + i + "value", OBJPROP_YDISTANCE, TableY + CellHeight * (i + 1)); ObjectSet("table_cell" + i + "value", OBJPROP_CORNER, 0); ObjectSet("table_cell" + i + "value", OBJPROP_BACK, true); ObjectSet("table_cell" + i + "value", OBJPROP_COLOR, TextColor); ObjectSet("table_cell" + i + "_value", OBJPROP_FONTSIZE, FontSize); } // Create vertical line ObjectCreate("vertical_line", OBJ_VLINE, 0, 0, 0); ObjectSet("vertical_line", OBJPROP_COLOR, TimeframeColor); ObjectSet("vertical_line", OBJPROP_WIDTH, 1); ObjectSet("vertical_line", OBJPROP_STYLE, STYLE_DASHDOT); // Set initial values CurrentPairIndex = 0; PreviousClosePrice = 0; PercentChange = 0; CurrentTimeframeColor = TimeframeColor; IsMovingVerticalLine = false; return(INIT_SUCCEEDED); } // Deinitialization function void OnDeinit(const int reason) { // Remove objects ObjectDelete("table_bg"); ObjectDelete("table_header"); for (int i = 0; i < 28; i++) { ObjectDelete("table_cell_" + i); ObjectDelete("table_cell_" + i + "_value"); } ObjectDelete("vertical_line"); } // Draw function void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { // Check for vertical line movement if (id == CHARTEVENT_OBJECT_DRAG && sparam == "vertical_line") { // Update vertical line position and percent change value double x = ObjectGet("vertical_line", OBJPROP_TIME1); int new_index = MathRound((ChartGetDouble(0, CHART_WIDTH_IN_PIXELS) - x - ChartGetInteger(0, CHART_XDISTANCE)) / ChartGetDouble(0, CHART_BARWIDTH)); if (new_index != CurrentPairIndex) { CurrentPairIndex = new_index; double close_price = SymbolInfoDouble(pairs[CurrentPairIndex], SYMBOL_CLOSE); PercentChange = (close_price - PreviousClosePrice) / PreviousClosePrice * 100; PreviousClosePrice = close_price; // Update table values for (int i = 0; i < ArraySize(pairs); i++) { // Check if pair label is selected if (ObjectGet("table_cell_" + i, OBJPROP_SELECTED)) { // Set color for selected pair ObjectSet("table_cell_" + i, OBJPROP_COLOR, SelectedPairColor); } else { ObjectSet("table_cell_" + i, OBJPROP_COLOR, TextColor); } // Update percent change value ObjectSetText("table_cell_" + i + "_value", DoubleToStr(SymbolInfoDouble(pairs[i], SYMBOL_CLOSE), Digits) + " (" + DoubleToStr((SymbolInfoDouble(pairs[i], SYMBOL_CLOSE) - SymbolInfoDouble(pairs[i], SYMBOL_PREVIOUS_CLOSE)) / SymbolInfoDouble(pairs[i], SYMBOL_PREVIOUS_CLOSE) * 100, 2) + "%)", CellWidth - 5, CellHeight, TL_RIGHT); } // Set color for selected pair ObjectSet("table_cell_" + i, OBJPROP_COLOR, SelectedPairColor); } else { ObjectSet("table_cell_" + i, OBJPROP_COLOR, TextColor); } // Update percent change value ObjectSetText("table_cell_" + i + "_value", DoubleToStr(SymbolInfoDouble(pairs[i], SYMBOL_CLOSE), Digits) + " (" + DoubleToStr((SymbolInfoDouble(pairs[i], SYMBOL_CLOSE) - SymbolInfoDouble(pairs[i], SYMBOL_PREVIOUS_CLOSE)) / SymbolInfoDouble(pairs[i], SYMBOL_PREVIOUS_CLOSE) * 100, 2) + "%)", CellWidth - 5, CellHeight, TL_RIGHT); } } ObjectSet("vertical_line", OBJPROP_TIME1, ChartTimeOnPosition(0, x)); ObjectSetText("vertical_line_percent_change", DoubleToStr(PercentChange, 2) + "%", 100, 20, TL_CENTER); } } // Custom indicator function int start() { // Do nothing return(0); }
It was simple. It couldn't have been clearer.
What part of “edit your original post” was unclear? What part of “DO NOT create a new post” was unclear? Why didn't you do the former, but instead did the latter?
Now, delete your post #4 and fix the original post and use the CODE button (or Alt+S)!
General rules and best pratices of the Forum. - General - MQL5 programming forum (2019)
Messages Editor
do you mean like this

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use