If you change the timeframe or the name of the chart symbol in MetaTrader, all indicators on the chart will be unloaded from the chart and will be loaded onto it again. Unlike MT4, in MT5 the sequence of load/unload is not defined due to its internal architecture.
This feature sometimes causes problems which are not immediately obvious. These problems are related to the fact that OnInit of the new loaded indicator instance can be executed before the OnDeinit of the instance being unloaded.
Situations in which this problem is detected, are most often connected with the desire to transmit (explicitly/implicitly) some information from OnDeinit of the older indicator instance to OnInit of the new one. In other words, the new indicator instance should know about the existence of the old one, and should not load until the old instance is unloaded.
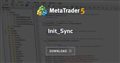
So OnDeinit() is called when the timeframe or symbol changes. I read that is the case but when I placed a Print("DeInit") in the OnDeInit function and changed timeframes, it does not print it at all. After including those libraries, it now does call the OnDeInit() and OnInit() functions in order. This should be the key to solving my problem. I'll work on it and post the solution when I'm finished. Thank you for your help! I never would have figured this out.
"prev_calculated not reset to 0 after timeframe change"
It's not true, is it?
Problem in OnInit()/OnDeinit() call sequence. The value of prev_calculated will be reset even if the sequence of calls is broken, right?
After all, the new copy of the indicator cannot know the value of prev_calculated for the old copy...
"prev_calculated not reset to 0 after timeframe change"
It's not true, is it?
Problem in OnInit()/OnDeinit() call sequence. The value of prev_calculated will be reset even if the sequence of calls is broken, right?
After all, the new copy of the indicator cannot know the value of prev_calculated for the old copy...
Right.
"prev_calculated not reset to 0 after timeframe change"
It's not true, is it?
Problem in OnInit()/OnDeinit() call sequence. The value of prev_calculated will be reset even if the sequence of calls is broken, right?
After all, the new copy of the indicator cannot know the value of prev_calculated for the old copy...
It's strange though because I had if(prev_calculated == 0 ) Print("prev_calculated = 0"); and it was only printing when I recompiled or first added it to the chart until I added those synch libraries. But I am skeptical now.
int OnCalculate(const int rates_total, const int prev_calculated, const int begin, const double& price[]) { while(GlobalVariableGet("new data")!=old_data && !IsStopped()){ ArrayInitialize(array,0); ArrayRemove(arrayBuffer,0,WHOLE_ARRAY); ReadFile(Filename); ArrayCopy(array,arrayBuffer,1,0, WHOLE_ARRAY ); old_data=GlobalVariableGet("new data"); } return(rates_total); }
Where do you check prev_calculated?
Why should I check though? I've just added a if(prev_calculated < rates_total) new_bar = true; But the WebRequest() takes about 2.7 seconds at its fastest to complete before the global variable is updated so I can't rely on tick data to know when to update the price array. Edit: I should mention, the data is never for the current bar, it only includes last completed bar.
Why should I check though? I've just added a if(prev_calculated < rates_total) new_bar = true; But the WebRequest() takes about 2.7 seconds at its fasted to complete before the global variable is updated so I can't rely on tick data to know when to update the price array.
I am confused by your reply.
You say that prev_calculated is not reset to zero when the TF is changed.
How do you Know? You don't check it in your code!
I am confused by your reply.
You say that prev_calculated is not reset to zero when the TF is changed.
How do you Know? You don't check it in your code!
Ah I see, sorry I removed the check from the code before I shared it. It was simply if(prev_calculated==0) Print("prev_calculated = 0"); And it wasn't printing when I changed timeframes but it is no matter, now it is working fine.
So I don't know why it wasn't printing, it must have been something wrong with my loop statement making it freeze or something.
Ok, here's my bitcoin aggregate volume indicator, not using an API but simply ( and slowly) downloading a csv file from bitcoinity.org. I am a beginner so if I did something that doesn't make sense, it's because I didn't know any better. But it works as I intend for the most part. Still needs a little cleaning up.
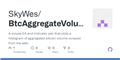
- SkyWes
- github.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
The code works unless I want to accumulate new bars. For example, the file data holds 50 bars of data, and with each new bar, the entire histogram plot is replaced with the new 50 bars of data, essentially shifting the data to the left to add a new bar. I don't want this. I want it to only update the entire array if the timeframe is changed. I've tried putting the condition of only updating the entire array if prev-calculated = 0 but that doesn't seem to reset to zero when the timeframe changes. I've tried to build it like a normal indicator, with arraysetasseries(false) and finding a starting point to fill the price[] using prev_calculated and rates_total and I can get both scenarios to work. The problem is when the timeframe changes, it breaks.
Any education would be appreciated. ps. Webrequest is so slow and this is not a very practical indicator for daytrading but it is a project I'm doing to challenge myself to better learn reading, cleaning, and transforming spreadsheets in code.