double rsi; double stochK; double stochD; double macdValue; double macdSignal; double atrValue; void OnTick() { rsi = iRSI(_Symbol, PERIOD_CURRENT, RSIPeriod, PRICE_CLOSE); stochK = iStochastic(_Symbol, PERIOD_CURRENT, StochKPeriod, StochDPeriod, MODE_EMA, 3, STO_LOWHIGH); stochD = iStochastic(_Symbol, PERIOD_CURRENT, StochKPeriod, StochDPeriod, MODE_EMA, 3, STO_LOWHIGH); macdValue = iMACD(_Symbol, PERIOD_CURRENT, MACDPeriod, MACDSignalPeriod, MACDHistogramPeriod, PRICE_CLOSE); macdSignal = iMACD(_Symbol, PERIOD_CURRENT, MACDPeriod, MACDSignalPeriod, MACDHistogramPeriod, PRICE_CLOSE);
Perhaps you should read the manual, especially the examples.
How To Ask Questions The Smart Way. (2004)
How To Interpret Answers.
RTFM and STFW: How To Tell You've Seriously Screwed Up.
They all (including iCustom) return a handle (an int). You get that in OnInit. In OnTick/OnCalculate (after the indicator has updated its buffers), you use the handle, shift and count to get the data.
Technical Indicators - Reference on algorithmic/automated trading language for MetaTrader 5
Timeseries and Indicators Access / CopyBuffer - Reference on algorithmic/automated trading language for MetaTrader 5
How to start with MQL5 - General - MQL5 programming forum - Page 3 #22 (2020)
How to start with MQL5 - MetaTrader 5 - General - MQL5 programming forum - Page 7 #61 (2020)
MQL5 for Newbies: Guide to Using Technical Indicators in Expert Advisors - MQL5 Articles (2010)
How to call indicators in MQL5 - MQL5 Articles (2010)
I have written a code with the following conditions:
Long:
-
RSI must be below the 50 line
-
Stochastic %K line must be above %D line
-
Stochastic %K line must be below 80
-
Enter when MACD line crosses above signal line
-
Set SL at twice the ATR below the entry candle low Set
-
TP at three times the ATR above the entry candle low
Short:
-
RSI must be above the 50 line
-
Stochastic %K line must be below %D line
-
Stochastic %D line must be above 20
-
Enter when MACD line crosses under signal line
-
Set SL at twice the ATR above the entry candle high
-
Set TP at three times the ATR below the entry candle high
The following is the code for the EA, and it doesnt seem to be opening any positions in the strategy tester:
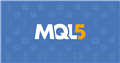
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have written a code with the following conditions:
Long:
RSI must be below the 50 line
Stochastic %K line must be above %D line
Stochastic %K line must be below 80
Enter when MACD line crosses above signal line
Set SL at twice the ATR below the entry candle low Set
TP at three times the ATR above the entry candle low
Short:
RSI must be above the 50 line
Stochastic %K line must be below %D line
Stochastic %D line must be above 20
Enter when MACD line crosses under signal line
Set SL at twice the ATR above the entry candle high
Set TP at three times the ATR below the entry candle high
The following is the code for the EA, and it doesnt seem to be opening any positions in the strategy tester: