It looks quite okay but I think you are making it way too complicated by using so many buffers. Try building an Indicator with one or two buffers and when that work take it from there... then you would have less error sources.
Question: Does it compile without errors?
Why did you set the buffers as series?
Tobias Johannes Zimmer #:
I have no error when i compile and it's not my indicator, i take it from the community. It looks quite okay but I think you are making it way too complicated by using so many buffers. Try building an Indicator with one or two buffers and when that work take it from there... then you would have less error sources.
Question: Does it compile without errors?
Why did you set the buffers as series? this is why it's difficult for me to modify this, and i just want to get the signal value with EMA smoothing.
Sorry if i don't speack properly but i'm not English.
But thanks for ur answer
SuzuA #:
I have no error when i compile and it's not my indicator, i take it from the community.
this is why it's difficult for me to modify this, and i just want to get the signal value with EMA smoothing.
Sorry if i don't speack properly but i'm not English.
But thanks for ur answer
I have no error when i compile and it's not my indicator, i take it from the community.
this is why it's difficult for me to modify this, and i just want to get the signal value with EMA smoothing.
Sorry if i don't speack properly but i'm not English.
But thanks for ur answer
Have a look at the documentation, they have an example there too
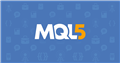
Documentation on MQL5: Technical Indicators / iMA
- www.mql5.com
iMA - Technical Indicators - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
I don't quite understand what your question is really about. MovingAverages.mqh is a file from the Include folder that is declared in the head of your code so its functions can be used.
#include <MovingAverages.mqh> #property indicator_separate_window #property indicator_buffers 6 .......... //--- calculate Signal buffer if(InpAppliedSignalMA==MODE_SMA) SimpleMAOnBuffer(rates_total,prev_calculated,0,InpSignalMA,ExtMacdBuffer,ExtSignalBuffer); if(InpAppliedSignalMA==MODE_EMA) ExponentialMAOnBuffer(rates_total,prev_calculated,0,InpSignalMA,ExtMacdBuffer,ExtSignalBuffer); if(InpAppliedSignalMA==MODE_SMMA) SmoothedMAOnBuffer(rates_total,prev_calculated,0,InpSignalMA,ExtMacdBuffer,ExtSignalBuffer); if(InpAppliedSignalMA==MODE_LWMA) LinearWeightedMAOnBuffer(rates_total,prev_calculated,0,InpSignalMA,ExtMacdBuffer,ExtSignalBuffer,weightsum_global);
The other thing is how can indicators be used in other programs, Expert Advisors:
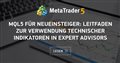
MQL5 für Neueinsteiger: Leitfaden zur Verwendung technischer Indikatoren in Expert Advisors
- www.mql5.com
Um Werte eines integrierten oder benutzerdefinierten Indikators in einem Expert Advisor zu erhalten, sollte zuerst sein Handle mithilfe der entsprechenden Funktion erstellt werden. Die Beispiele in diesem Beitrag zeigen, wie diese und jene technischen Indikatoren während der Erstellung Ihrer eigenen Programme genutzt werden können. Dieser Beitrag beschreibt Indikatoren, die in MQL5 geschrieben werden. Er richtet sich an jene, die nicht viel Erfahrung in der Entwicklung von Handelsstrategien haben, und liefert einfache und klare Arten der Arbeit mit Indikatoren mithilfe der bereitgestellten Bibliothek von Funktionen.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
curve, can someone explain me how ?