With the help of Alain's code
https://www.mql5.com/en/forum/321205
and chatgpt :) this works on MT5 for me (version 5 build 3559):
#include <WinAPI\winuser.mqh> #include <WinAPI\winbase.mqh> #define GMEM_MOVEABLE 0x0002 #define CF_UNICODETEXT 13 #import "kernel32.dll" string lstrcpyW(PVOID string1,const string string2); string lstrcpyW(string string1,PVOID string2); #import string GetTextFromClipboard() { string Text = ""; // Try grabbing ownership of the clipboard if(OpenClipboard(0)!=0) { // Try getting the clipboard data HANDLE hClipboardData = GetClipboardData(CF_UNICODETEXT); if(hClipboardData != 0) { // Try locking the memory, so that we can copy from it PVOID ptrMem = GlobalLock(hClipboardData ); if(ptrMem != 0) { // Copy the string from the global memory Text = lstrcpyW(Text, ptrMem); // Release ownership of the global memory GlobalUnlock(hClipboardData); } } // Always release the clipboard, even if the copy failed CloseClipboard(); } return (Text); } void OnStart() { string test = GetTextFromClipboard(); }
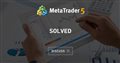
I solved this problem with this change:
int GetClipboardData(int uFormat);
=>
long GetClipboardData(int uFormat);

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello
I use this script for get text from windows clipboard.
When copy "HELLO" to clipboard, function returns a big number like 19522312522325488
How can I fix this code or convert this number to "HELLO"?
Thanks