Milan Zivanovic: I want to know how many times "USD" is repeated in this list.
So go through the list and count them. What's the problem?
Alexandre Borela #:
How are you calling ArrayValCnt? What values are you passing to it? Because in the first post,
the list you posted is not an array.
maybe I wasn’t clear enough at first post. I wanted to make it as simple as possible. It can be array or list, I tried both.
int ArrayValCnt(string& array[], string val) { int cnt = 0; for(int z=0;z<ArraySize(array);z++) { if(StringFind(array[z],val)>-1) cnt++; } return(cnt); }Pairs = "EURUSD,GBPUSD,GBPUSD,USDCHF,USDJPY"; StringSplit(Pairs,',',TradePairs); for(int i=0; i<ArraySize(TradePairs); i++) { ArrayValCnt(TradePairs[i], "USD"); }
Milan Zivanovic #:
I see the error. You first split string which means your ArrayValCnt only see only 1 element. Call it directly on the split string:maybe I wasn’t clear enough at first post. I wanted to make it as simple as possible. It can be array or list, I tried both.
Pairs = "EURUSD,GBPUSD,GBPUSD,USDCHF,USDJPY"; StringSplit(Pairs,',',TradePairs); int usdCount = ArrayValCnt(TradePairs, "USD");
//+------------------------------------------------------------------+ //| StringCountMatches. | //+------------------------------------------------------------------+ /** * Counts the number of all occurrences of the specified string in * the input string using case-sensitive search. * Example: * string str = "Mr Blue has a blue house and a blue car"; * Print( StringCountMatches(str, "blue") ); // 2 */ int StringCountMatches(string sourceStr, string searchStr) { int count = StringReplace(sourceStr, searchStr, "");; return count; } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnStart() { string Pairs = "EURUSD,GBPUSD,GBPUSD,USDCHF,USDJPY"; int count = StringCountMatches(Pairs, "USD"); Print("Count of matches: ", count); } //+------------------------------------------------------------------+ // Count of matches: 5
String Manipulation Functions - library
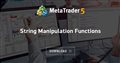
This solution is far worse than the one OP posted with StringFind as you will generate unnecessary temporary strings.
//+------------------------------------------------------------------+ //| StringCountMatches. | //+------------------------------------------------------------------+ /** * Counts the number of all occurrences of the specified string in * the input string using case-sensitive search. * Example: * string str = "Mr Blue has a blue house and a blue car"; * Print( StringCountMatches(str, "blue") ); // 2 */ int StringCountMatches(string sourceStr, string searchStr) { // return StringReplace(sourceStr, searchStr, ""); int count = 0; int pos = 0; while((pos = StringFind(sourceStr, searchStr, pos)) != -1) { count++; pos += StringLen(searchStr); } return count; }

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
I have a list of symbols.
Pairs = "EURUSD,GBPUSD,GBPUSD,USDCHF,USDJPY";
I want to know how many times "USD" is repeated in this list.
Pairs = "EURUSD,GBPUSD,GBPUSD,USDCHF,USDJPY";
I want to know this for others as well, for example for "GBP" and "EUR".