How is it possible that the alert "total trades" is returning '3', but "have we had two trades?" is returning 'false'. This makes no sense... my head hurts. Please help.
You are checking if it is BIGGER OR equals:
if(totalTrades >= 2) haveWeHadTwoTrades=true;
If you want to check if its exactly 2, you have to use ==:
if(totalTrades == 2) haveWeHadTwoTrades=true;
Read the documentation or some tutorials first before coding or you'll encounter many issues like this along the road:
https://www.mql5.com/en/docs/basis/operations/relation
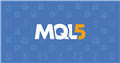
- www.mql5.com
dreaming MT6 with easy language...
It's a balance, if a language is too simplistic then it would prevent the programmers from doing complex stuff,
I'm glad they designed it like C++, although is not easy for beginner developers, it gives those that know how
to code unlimited potential and I still miss some C++'s features like metaprograming.
-
if(totalTrades >= 2) haveWeHadTwoTrades==true;
haveWeHadTwoTrades has no initial value; result meaningless. -
That's not an assignment; bool value not used.
-
Compute your result after you count all orders.
bool haveWeHadTwoTrades=totalTrades >= 2;
It's a balance, if a language is too simplistic then it would prevent the programmers from doing complex stuff,
I'm glad they designed it like C++, although is not easy for beginner developers, it gives those that know how
to code unlimited potential and I still miss some C++'s features like metaprograming.
yeah but there are some who just want to be traders and not expert programmers...for me it should maintain mql language as you said you can do everything, but it should integrate easy language scripts, in fact there are platforms that were not born with easy language but it has been integrated.
They are already doing this, there's python:
https://www.mql5.com/en/docs/integration/python_metatrader5
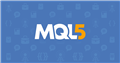
- www.mql5.com
You are checking if it is BIGGER OR equals:
If you want to check if its exactly 2, you have to use ==:
Read the documentation or some tutorials first before coding or you'll encounter many issues like this along the road:
https://www.mql5.com/en/docs/basis/operations/relation
Thanks for everyone's comments, here's the solution I used:
bool HaveWeHadTwoTrades(int Magic_Number, ENUM_TIMEFRAMES Validation_TimeFrame) { int totalTrades; for(int i = OrdersHistoryTotal()-1 ; i >= 0 ; i--) { if(OrderSelect(i,SELECT_BY_POS,MODE_HISTORY)) { if(OrderSymbol() == Symbol() && OrderMagicNumber() == Magic_Number && OrderOpenTime() > iTime(Symbol(),Validation_TimeFrame,0)) totalTrades = totalTrades + 1; } } if(totalTrades == 2) return true; return false; }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
How is it possible that the alert "total trades" is returning '3', but "have we had two trades?" is returning 'false'. This makes no sense... my head hurts. Please help.