The main mistake: you create an indicator handle at every tick.
REMEMBER: IN MQL5, the indicator handle MUST BE CREATED ONCE! And this is done in OnInit !!!
Vladimir Karputov:
Thanks for the response Vlad! Any recommendation for where to move the code? I tried moving the handles to OnInit but got an error stating the variable is undefined.
The main mistake: you create an indicator handle at every tick.
REMEMBER: IN MQL5, the indicator handle MUST BE CREATED ONCE! And this is done in OnInit !!!
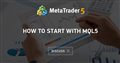
How to start with MQL5
- 2020.09.17
- www.mql5.com
This thread discusses MQL5 code examples. There will be examples of how to get data from indicators, how to program advisors...
Thanks Vlad, your examples showed me another major problem with my code. I was under the impression buy and sell commands worked in tandem with eachother, clearly thats not the case and all the positions being triggered were staying open, I need to make sure they are closed before a new order is opened.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi everyone, I'm new to the MT platform and so far I really like it. I've been developing my first advisor but I'm running into an issue.
I pieced this script together from resources I found around the internet. I have basic coding skills but I'm certainly not a pro, so please excuse me if I'm making a glaring mistake.
Essentially the issue is that the advisor keeps making trades on every single candle if the condition is met. I only want it to execute a buy once when the opening price crosses above the "confimation" SMA line, and sell when the asking price crosses below the "validation" SMA line.
I figured the best way to go about it would be to check the previous bar to make sure its not placing a new order higher than the previous one, but I'm not sure how to go about it.
Any help would be greatly appreciated!