First, fix the main mistake: you create an indicator on every tick.
Remember: in MQL5, the indicator handle is created ONCE !!! And this is done in OnInit !!!
First, fix the main mistake: you create an indicator on every tick.
Remember: in MQL5, the indicator handle is created ONCE !!! And this is done in OnInit !!!
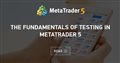
- www.mql5.com
Sorry, but I didn't understand. I created na indicator on every tick? How's that? How do I solve it?
First, fix the main mistake: you create an indicator on every tick.
Remember: in MQL5, the indicator handle is created ONCE !!! And this is done in OnInit !!!
Ohhhh now I did understand. So, I need to move all my code minus the if, to void onInit() right?
Ohhhh now I did understand. So, I need to move all my code minus the if, to void onInit() right?
An example of get values from the iRSI indicator
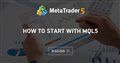
- 2020.07.05
- www.mql5.com
Your code checks for 70 or above and opens. Where do you check if a trade is already open?
I corrected my main mistake about the indicator handle, but now it creates two long trades at a time. How do I check if a trade is already open?
#include<Trade/trade.mqh> CTrade trade; int myRSIDefinition; int OnInit() { //defining the properties of the RSI myRSIDefinition = iRSI(_Symbol, _Period, 14, PRICE_CLOSE); return(INIT_SUCCEEDED); } void OnTick() { //creating an array for several prices double myRSIArray[]; //sorting the price array from the current candle downwards ArraySetAsSeries(myRSIArray,true); //defined EA, current candle, 3 candles, store result CopyBuffer(myRSIDefinition, 0, 0, 3, myRSIArray); //calculating RSI for the current candle double myRSIValue= NormalizeDouble(myRSIArray[0],2); //if he value of the RSI is greater than 70, then the EA opens a long trade if(myRSIValue<70) { double Ask=NormalizeDouble(SymbolInfoDouble(_Symbol, SYMBOL_ASK),_Digits); double Balance= AccountInfoDouble(ACCOUNT_BALANCE); double Equity= AccountInfoDouble(ACCOUNT_EQUITY); if(Equity >= Balance){ trade.Buy(0.05, NULL, Ask,0,(Ask+100 * _Point), NULL); } }else{ } }
I think you re trying to open only 1 order for every candle, if so this piece of code will help you;
bool newBar() { static datetime TimeBar=0; bool flag=false; if(TimeBar!=iTime(Symbol(),Period(),0)) { TimeBar=iTime(Symbol(),PERIOD_CURRENT,0); flag=true; } // return true if you are in new bar. return (flag); }
if you want to wait until your open position close to place new order, you can simply
if(PositionsTotal() < 1)
Check if there is an open position before placing new one.
Hope these would help
You can create a function to calculate current total orders.
Or just simply check using OrdersTotal() function like this
I think you re trying to open only 1 order for every candle, if so this piece of code will help you;
if you want to wait until your open position close to place new order, you can simply
Check if there is an open position before placing new one.
Hope these would help

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm trying to make my EA to open a single long trade ONLY when it crosses the 70 level. Unfortunately, it opens many buy orders. I did something wrong but I can't find the error.