Hi everyone,
I am trying to draw a standard deviation channel as part of my EA using the Least Squares Method: https://www.mathsisfun.com/data/least-squares-regression.html
But I am getting some strange values. Can anyone spot something in the code that I am doing wrong? Or is it just because iTime and iClose are real time values and that I am getting random errors when calling them?
The code below creates the white line in the attached screenshot. This line is suppose to be the same as the center line of the red standard deviation channel in the image
Hi I've looked through and the maths looks okay assuming your MathSum function is correct and all the data types are correct.
However, you might try the following:
- use the index number for the X axis instead of time, that way you will avoid any casting issues
- do not use ArraySetAsSeries it is not necessary
- store the points in X ascending order as that is how the x-axis works so X[0] = 1 X[1] = 20 X[2] = 40 and the corresponding Y values in same order
- substitute the real index time when you plot the line at the end
- make sure you use type double for everything except X can be an integer and the index time for the object plot should be datetime
Hi I've looked through and the maths looks okay assuming your MathSum function is correct and all the data types are correct.
However, you might try the following:
- use the index number for the X axis instead of time, that way you will avoid any casting issues
- do not use ArraySetAsSeries it is not necessary
- store the points in X ascending order as that is how the x-axis works so X[0] = 1 X[1] = 20 X[2] = 40 and the corresponding Y values in same order
- substitute the real index time when you plot the line at the end
- make sure you use type double for everything except X can be an integer and the index time for the object plot should be datetime
Hi Paul,
Thank you for taking the time to look through the code.
The MathSum that I am using is the subclass in the standard library.
I will work on those suggestions and get back to you.
Regards
Jan-Harm
I did the MathSum calculations and it turned out that the function does not work correctly. As soon as I assigned the values that I calculated by hand everything worked.
Any idea why the MathSum is not working?
https://www.mql5.com/en/docs/standardlibrary/mathematics/stat/mathsubfunctions/statmathsum
Update: It seems that a random value is added to the Arrays at the [3] position even though I am not assigning something to it. So because the MathSum function is looking at the whole array it is considering the [3] position as well.
If I try and limit the array size to 3 ([0],[1] and [2]) the EA removes itself from the chart.
//Ensures that all arrays have "enough space" ArrayResize(TrendX_Array,3,0); ArrayResize(TrendY_Array,3,0); ArrayResize(TrendXsqr_Array,3,0); ArrayResize(TrendXY_Array,3,0); ArrayResize(AnchorClose,3,0); ArrayResize(AnchorTime,3,0);
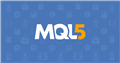
- www.mql5.com
I did the MathSum calculations and it turned out that the function does not work correctly. As soon as I assigned the values that I calculated by hand everything worked.
Any idea why the MathSum is not working?
https://www.mql5.com/en/docs/standardlibrary/mathematics/stat/mathsubfunctions/statmathsum
Update: It seems that a random value is added to the Arrays at the [3] position even though I am not assigning something to it. So because the MathSum function is looking at the whole array it is considering the [3] position as well.
If I try and limit the array size to 3 ([0],[1] and [2]) the EA removes itself from the chart.
Ok that is understandable, MQL does not initialise anything by default, so you can get random values in newly declared variables. You could simply fill the arrays with zero at the start to solve that.
Why the EA closes I can't guess at without all the code, try checking the Experts tab for error messages.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi everyone,
I am trying to draw a standard deviation channel as part of my EA using the Least Squares Method: https://www.mathsisfun.com/data/least-squares-regression.html
But I am getting some strange values. Can anyone spot something in the code that I am doing wrong? Or is it just because iTime and iClose are real time values and that I am getting random errors when calling them?
The code below creates the white line in the attached screenshot. This line is suppose to be the same as the center line of the red standard deviation channel in the image