
You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Minimum code:
Minimum code:
Thank you, so much. Solved the problem.
But my entry and exit not work,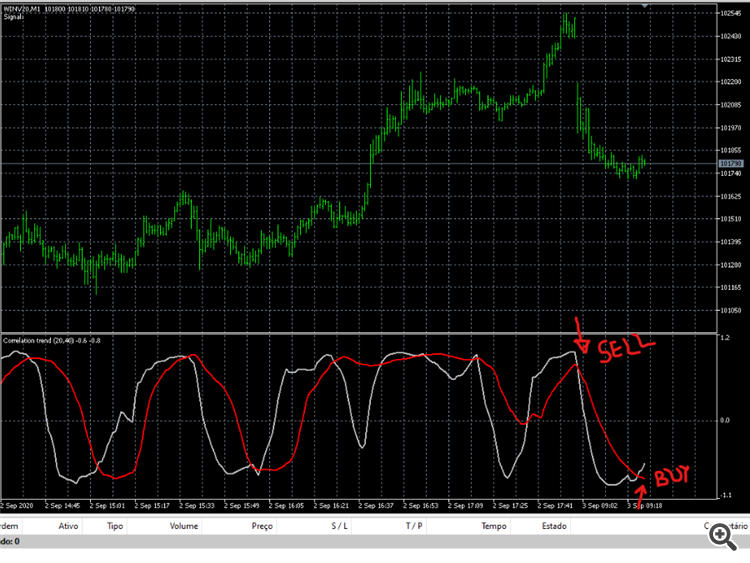
Thank you, so much. Solved the problem.
But my entry and exit not work,
Anexe ( ) dois arquivos MQ5 (SOMENTE MQ5): o arquivo indicador e o arquivo FIXED advisor.
I Can´t find Corola.mq5
I Can´t find Corola.mq5
If you want help - Upload MQ5 files - I'm not going to download and run the CLOSED file.
Se você quiser ajuda - Carregar arquivos MQ5 - não vou baixar e executar o arquivo CLOSED .
Sorry, I found.
Sorry, I found.
Indicator 'Corola.mq5'
Expert advisor 'Coro.mq5'