Check out the article The checks a trading robot must pass before publication in the Market
Read especially about the freeze levels ...
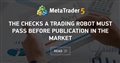
- www.mql5.com
Check out the article The checks a trading robot must pass before publication in the Market
Read especially about the freeze levels ...
Thanks. Was quite interesting. didn't know that these existed. However it did not solve my problem. the stop and freeze level where 0.
i have modified the code to check the stop and freeze levels:
STP (0.00195) and TKP (0.0156) are global variables that hold the stop loss and take profit in pips.
bid = 1.3426
void MakeTrade(string trade_type, double lots) { MqlTick latest_price; double trade_SL, trade_TP, trade_BE, trade_open_price; string trade_comment; int stops_level = (int)SymbolInfoInteger(_Symbol, SYMBOL_TRADE_STOPS_LEVEL); if(stops_level != 0) { PrintFormat("SYMBOL_TRADE_STOPS_LEVEL=%d: StopLoss and TakeProfit must" + " not be nearer than %d points from the closing price", stops_level, stops_level); //check stops if (STP > stops_level * _Point) { Alert("##FK## !!!!!!!! ", trade_type, " Stop Loss > Stop Level"); return; } if (TKP > stops_level * _Point) { Alert("##FK## !!!!!!!! ", trade_type, " Take Profit > Stop Level"); return; } } int freeze_level = (int)SymbolInfoInteger(_Symbol, SYMBOL_TRADE_FREEZE_LEVEL); if(freeze_level != 0) { PrintFormat("SYMBOL_TRADE_FREEZE_LEVEL=%d: StopLoss and TakeProfit must" + " not be nearer than %d points from the closing price", freeze_level, freeze_level); //check stops if (STP > freeze_level * _Point) { Alert("##FK## !!!!!!!! ", trade_type, " Stop Loss > Freeze Level"); return; } if (TKP > freeze_level * _Point) { Alert("##FK## !!!!!!!! ", trade_type, " Take Profit > Freeze Level"); return; } } //--- Get the last price quote using the MQL5 MqlTick Structure if(!SymbolInfoTick(_Symbol, latest_price)) { Alert("Error getting the latest price quote - error:", GetLastError(), "!!"); return; } if (trade_type == "BUY") { trade_open_price = NormalizeDouble(latest_price.ask, _Digits); trade_SL = NormalizeDouble(trade_open_price - STP, _Digits); trade_TP = NormalizeDouble(trade_open_price + TKP, _Digits); trade_BE = NormalizeDouble(trade_open_price + BE_Val, _Digits); } else if (trade_type == "SELL") { trade_open_price = NormalizeDouble(latest_price.bid, _Digits); trade_SL = NormalizeDouble(trade_open_price + STP, _Digits); trade_TP = NormalizeDouble(trade_open_price - TKP, _Digits); trade_BE = NormalizeDouble(trade_open_price - BE_Val, _Digits); } trade_comment = StringFormat("%s %s %G lots at %s, SL=%s SLP=%s TP=%s TPP=%s", trade_type, _Symbol, lots, DoubleToString(trade_open_price, _Digits), DoubleToString(trade_SL, _Digits), DoubleToString(trade_SL - trade_open_price, _Digits), DoubleToString(trade_TP, _Digits), DoubleToString(trade_TP - trade_open_price, _Digits)); if (trade_type == "BUY") { if(!trade.Buy(lots, _Symbol, trade_open_price, trade_SL, trade_TP, trade_comment)) { //--- failure message Print("#### !!!!!!!! ", trade_type, " Trade method failed. Return code=", trade.ResultRetcode(), ". Code description: ", trade.ResultRetcodeDescription()); Alert("#### ", trade_type, " Trade method failed. Return code=", trade.ResultRetcode(), ". Code description: ", trade.ResultRetcodeDescription()); return; } else { Print(trade_type, " Trade method executed successfully. Return code=", trade.ResultRetcode(), " (", trade.ResultRetcodeDescription(), ")"); Print("#### ", trade_comment); } } else if (trade_type == "SELL") { if(!trade.Sell(lots, _Symbol, trade_open_price, trade_SL, trade_TP, trade_comment)) { //--- failure message Print("#### !!!!!!!!! ", trade_type, " Trade method failed. Return code=", trade.ResultRetcode(), ". Code description: ", trade.ResultRetcodeDescription()); Alert("#### ", trade_type, " Trade method failed. Return code=", trade.ResultRetcode(), ". Code description: ", trade.ResultRetcodeDescription()); return; } else { Print("#### ", trade_type, " Trade method executed successfully. Return code=", trade.ResultRetcode(), " (", trade.ResultRetcodeDescription(), ")"); Print("#### ", trade_comment); } } else { return; } }
Thanks. Was quite interesting. didn't know that these existed. However it did not solve my problem. the stop and freeze level where 0.
i have modified the code to check the stop and freeze levels:
STP (0.00195) and TKP (0.0156) are global variables that hold the stop loss and take profit in pips.
bid = 1.3426
Check the spread at the time of the error. Even better - if there is an error - print the Ask and Bid.
Check the spread at the time of the error. Even better - if there is an error - print the Ask and Bid.
Ah yes that did it.
SL > ask
again didn't know that was a thing.
thanks Vladimir
Check out the article The checks a trading robot must pass before publication in the Market
Read especially about the freeze levels ...
Vladimir every where...
Thanks a man... that solved my problem :)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi,
I am building an EA to automate trading. i have a method that enters into a trade. it seems to work most of the time, however i get the following error at times:
2020.08.27 15:30:50.195 2017.12.29 00:00:00 failed instant sell 0.03 GBPUSD at 1.34260 sl: 1.34455 tp: 1.32700 [Invalid stops]
2020.08.27 15:30:50.195 2017.12.29 00:00:00 CTrade::OrderSend: instant sell 0.03 GBPUSD at 1.34260 sl: 1.34455 tp: 1.32700 [invalid stops]
i don't understand what is wrong with the stops here. sell trade so sl is higher then price and tp is lower then price.
code below. any help would be appreciated.
thanks