Hi
My query is , " how to calculate price of the signal candle?"
Can any one help me please?
Below is my code for indicator.
Please use the 'Code' button .
I want to show the opening candle price of signal candle, how to do it please..
Forum on trading, automated trading systems and testing trading strategies
how to calculate price of the signal candle
Vladimir Karputov, 2020.08.03 12:31
Please use the 'Code' button .
I dint get you, can you please clarify,,
Paste your indicator code into messages. Use the button to insert code.
By submitting code, how my query will get solved.??
By submitting code, how my query will get solved.??
Sorry, all of our wizards and telapats are on vacation right now. Without them, We cannot read your thoughts. There is only one way left: you must attach the code of your indicator using the button .
Hi
Below is my code for indicator.
there is nothing below!
there is nothing below!
If you talking about the Bid / Ask Price of the signal at the moment that signal candle alert comes out then you need to put something in your code to output to your alert.
Something like:
Print(Ask) or Print(Buy) to give you the price at that moment.
Then use that data to put it where ever you need it or send it to your alert as well.
Anyhow just what little I know and that is not much.
Here is docs.
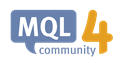
- docs.mql4.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi
My query is , " how to calculate price of the signal candle?"
Can any one help me please?
Below is my code for indicator.