Inputs have to be declared on a global scope (outside OnStart()).
#property script_show_inputs //--- Hours enum Hours { H8H00=0, //8:00 H9H00=1, //9:00 H10H00=2, //10:00 }; //--- input parameters input Hours swapHour = H8H00; //--- Bias enum Bias { Buy=0, //Buy BAG=1, //BAG BAHG=2, //BAHG }; //--- input parameters input Bias swapBias = Buy; //+------------------------------------------------------------------+ //| script program start function | //+------------------------------------------------------------------+ void OnStart() { int corner = 1; //0 - for top-left corner, 1 - top-right, 2 - bottom-left, 3 - bottom-right int x = 20; int y = 380; ObjectDelete(NULL,"H1_Bias"); //---- ObjectCreate(NULL,"H1_Bias",OBJ_LABEL,0,0,0); ObjectSet("H1_Bias", OBJPROP_CORNER, corner); ObjectSet("H1_Bias", OBJPROP_XDISTANCE, x); ObjectSet("H1_Bias", OBJPROP_YDISTANCE, y); ObjectSet("H1_Bias", OBJPROP_BACK, true); ObjectSet("H1_Bias", OBJPROP_SELECTABLE, false); ObjectSetText("H1_Bias",StringSubstr(EnumToString(swapHour),1) + " " + EnumToString(swapBias),12,"Arial",clrSnow); }
Thanks for your reaction Ernst, I will see if I can get this working.
In the mean time I made a version with input by hand, but I would still like to have the drop down menu.
Best regards,
Robert
I get the error message : " 'input' - unexpected token" so my understanding of how this should be done is not right.
You should show your code where the error is reported.
Probably you are missing the ";" from the line previous to the error reported.
I managed to get the code without errors, but the options from the enumeration are not shown when I activate the script. The input tab just shows Variable: "swapBias" with the Value: "0", but it does not show the options for the other values.
Attached the latest script, could someone please tell me what the correct code should be in order to show the options in the input tab?
//--- Bias enum Bias { B1=0, //"Buy"; B2=1, //"BAG"; B3=2, //"BAHG"; B4=3, //"BEMA"; B5=4, //"Sell"; B6=5, //"SBG"; B7=6, //"SBHG"; B8=7, //"SEMA"; B9=8, //"WFC"; B10=9, //"WFD"; }; //--- input parameters static input Bias Bias_1 = "Buy"; static input Bias Bias_2 = 1; static input Bias Bias_3 = 2; static input Bias Bias_4 = "BEMA"; static input Bias Bias_5 = "Sell"; static input Bias Bias_6 = "SBG"; static input Bias Bias_7 = "SBHG"; static input Bias Bias_8 = "SEMA"; static input Bias Bias_9 = "WFC"; static input Bias Bias_10 = "WFD";
I probably got it wrong again i didn't test it
static input Bias Bias_1 = B1;
Thanks Marco and thanks William.
I made a new version with your input, but compile generates two types of errors.
static input Bias Bias_1 = B1; static input Bias Bias_2 = B2; static input Bias Bias_3 = B3; static input Bias Bias_4 = B4; static input Bias Bias_5 = B5; static input Bias Bias_6 = B6; static input Bias Bias_7 = B7; static input Bias Bias_8 = B8; static input Bias Bias_9 = B9; static input Bias Bias_10 = B10;
every line of the above code has the error: 'input' - unexpected token
and each of the lines below has the error: '=' - name expected
if(swapBias == B1) {Bias = Bias_1;} if(swapBias == B2) {Bias = Bias_2;} if(swapBias == B3) {Bias = Bias_3;} if(swapBias == B4) {Bias = Bias_4;} if(swapBias == B5) {Bias = Bias_5;} if(swapBias == B6) {Bias = Bias_6;} if(swapBias == B7) {Bias = Bias_7;} if(swapBias == B8) {Bias = Bias_8;} if(swapBias == B9) {Bias = Bias_9;} if(swapBias == B10) {Bias = Bias_10;}
I don't know what you want to do.
I had hoped you would fiddle with it until it worked but some elements are displaced.
//+------------------------------------------------------------------+ //| Bias.mq4 | //| Copyright 2019, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2019, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property strict //input int Bias; input int swapBias; //--- Bias enum Bias { B1=0, //"Buy"; B2=1, //"BAG"; B3=2, //"BAHG"; B4=3, //"BEMA"; B5=4, //"Sell"; B6=5, //"SBG"; B7=6, //"SBHG"; B8=7, //"SEMA"; B9=8, //"WFC"; B10=9, //"WFD"; }; //--- input parameters static input Bias Bias_1 = 0; static input Bias Bias_2 = 1; static input Bias Bias_3 = 2; static input Bias Bias_4 = 3; static input Bias Bias_5 = 4; static input Bias Bias_6 = 5; static input Bias Bias_7 = 6; static input Bias Bias_8 = 7; static input Bias Bias_9 = 8; static input Bias Bias_10 = 9; int Bias1,Bias2,Bias3,Bias4,Bias5,Bias6,Bias7,Bias8,Bias9,Bias10; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- if(swapBias == 0) {Bias1=0;} if(swapBias == 1) {Bias2=1;} if(swapBias == 2) {Bias3=2;} if(swapBias == 3) {Bias4=3;} if(swapBias == 4) {Bias5=4;} if(swapBias == 5) {Bias6=5;} if(swapBias == 6) {Bias7=6;} if(swapBias == 7) {Bias8=7;} if(swapBias == 8) {Bias9=8;} if(swapBias == 9) {Bias10=9;} //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+
//+------------------------------------------------------------------+ //| Swap_Bias.mq4 | //| Copyright 2019 , Lorentzos Roussos | //| https://www.mql5.com/en/users/lorio | //+------------------------------------------------------------------+ #property copyright "Copyright 2019 , Lorentzos Roussos" #property link "https://www.mql5.com/en/users/lorio" #property version "1.00" #property strict #property script_show_inputs input string sSwapTime="00:00:00";//Swap Time enum bias_types { bias_buy=0,//"Buy" bias_BAG=1,//"BAG" bias_BAHG=2,//"BAHG" bias_Sell=3,//"Sell" bias_SBG=4,//"SBG" bias_SBHG=5,//"SBHG" bias_WFC=6,//"WFC" bias_WFD=7//"WFD" }; input bias_types Bias=bias_buy;//Bias : //Display Array string bias_text[]={"Buy","BAG","BAHG","Sell","SBG","SBHG","WFC","WFD"}; string swap_modes[]={"Disabled","Points","Currency Symbol","Currency Margin","Currency Deposit","Interest Current","Interest Open","Reopen Current","Reopen Bid"}; //time keeper struct simple_time { string represent; int hour; int minute; int second; }; simple_time SwapTime; enum symbol_swap_mode { swap_mode_disabled=0,//DISABLED swap_mode_points=1,//POINTS swap_mode_currency_symbol=2,//CURRENCY SYMBOL swap_mode_currency_margin=3,//CURRENCY MARGIN swap_mode_currency_deposit=4,//CURRENCY DEPOSIT swap_mode_interest_current=5,//INTEREST CURRENT swap_mode_interest_open=6,//INTEREST OPEN swap_mode_reopen_current=7,//REOPEN CURRENT swap_mode_reopen_bid=8//REOPEN BID }; symbol_swap_mode ssm; string system_tag="SwBi_"; //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- ObjectsDeleteAll(0,system_tag); ssm=(symbol_swap_mode)SymbolInfoInteger(Symbol(),SYMBOL_SWAP_MODE); SwapTime=StringToSimpleTime(sSwapTime); int x=10; int y=10; int ystep=20; int fontsize=12; string font="Arial"; color text_color=clrWhite; color back_color=clrRoyalBlue; color border_color=clrMidnightBlue; CreateRectangle(0,0,system_tag+"_back",NULL,x,y,120,100,back_color,border_color,BORDER_RAISED,false,false); x=20; y+=ystep; CreateLabel(0,0,system_tag+"_swap_mode","Swap Mode",x,y,0,0,font,fontsize,swap_modes[ssm],text_color,ALIGN_LEFT,ANCHOR_LEFT,false,clrNONE,clrNONE,BORDER_FLAT,false,false); y+=ystep; CreateLabel(0,0,system_tag+"_bias","Bias",x,y,0,0,font,fontsize,bias_text[Bias],text_color,ALIGN_LEFT,ANCHOR_LEFT,false,clrNONE,clrNONE,BORDER_FLAT,false,false); y+=ystep; CreateLabel(0,0,system_tag+"_swap_time","Swap Time",x,y,0,0,font,fontsize,SwapTime.represent,text_color,ALIGN_LEFT,ANCHOR_LEFT,false,clrNONE,clrNONE,BORDER_FLAT,false,false); } //+------------------------------------------------------------------+ simple_time StringToSimpleTime(string string_time) { simple_time returnio; ushort usep=StringGetCharacter(":",0); string result[]; returnio.represent="00:00:00"; returnio.hour=0; returnio.minute=0; returnio.second=0; int k=StringSplit(string_time,usep,result); for(int i=0;i<k;i++) { if(i==0) returnio.hour=(int)StringToInteger(result[i]); if(i==1) returnio.minute=(int)StringToInteger(result[i]); if(i==2) returnio.second=(int)StringToInteger(result[i]); } string hours_rep=IntegerToString(returnio.hour); if(StringLen(hours_rep)<2) hours_rep="0"+hours_rep; string mins_rep=IntegerToString(returnio.minute); if(StringLen(mins_rep)<2) mins_rep="0"+mins_rep; string secs_rep=IntegerToString(returnio.second); if(StringLen(secs_rep)<2) secs_rep="0"+secs_rep; returnio.represent=hours_rep+":"+mins_rep+":"+secs_rep; ArrayFree(result); return(returnio); } void CreateRectangle(long chart_id, int subwindow, string name, string tooltip, int px, int py, int sx, int sy, color back_color, color border_color, ENUM_BORDER_TYPE border_type, bool back, bool selectable) { bool obji=ObjectCreate(chart_id,name,OBJ_RECTANGLE_LABEL,subwindow,0,0); ObjectSetInteger(chart_id,name,OBJPROP_BORDER_TYPE,border_type); ObjectSetInteger(chart_id,name,OBJPROP_BORDER_COLOR,border_color); ObjectSetInteger(chart_id,name,OBJPROP_BGCOLOR,back_color); ObjectSetInteger(chart_id,name,OBJPROP_XSIZE,sx); ObjectSetInteger(chart_id,name,OBJPROP_YSIZE,sy); ObjectSetInteger(chart_id,name,OBJPROP_XDISTANCE,px); ObjectSetInteger(chart_id,name,OBJPROP_YDISTANCE,py); ObjectSetInteger(chart_id,name,OBJPROP_BACK,back); ObjectSetInteger(chart_id,name,OBJPROP_SELECTABLE,selectable); ObjectSetString(chart_id,name,OBJPROP_TOOLTIP,tooltip); } void CreateLabel(long chart_id, int subwindow, string name, string tooltip, int px, int py, int sx, int sy, string font, int fontsize, string text, color txt_color, ENUM_ALIGN_MODE align, ENUM_ANCHOR_POINT anchor, bool with_rectangle, color back_col, color border_col, ENUM_BORDER_TYPE border_type, bool selectable, bool back) { bool obji=false; if(with_rectangle) ObjectCreate(chart_id,name,OBJ_EDIT,subwindow,0,0); if(!with_rectangle) ObjectCreate(chart_id,name,OBJ_LABEL,subwindow,0,0); ObjectSetString(chart_id,name,OBJPROP_TEXT,text); ObjectSetString(chart_id,name,OBJPROP_TOOLTIP,tooltip); ObjectSetString(chart_id,name,OBJPROP_FONT,font); ObjectSetInteger(chart_id,name,OBJPROP_ALIGN,align); ObjectSetInteger(chart_id,name,OBJPROP_ANCHOR,anchor); ObjectSetInteger(chart_id,name,OBJPROP_FONTSIZE,fontsize); if(with_rectangle) ObjectSetInteger(chart_id,name,OBJPROP_XSIZE,sx); if(with_rectangle) ObjectSetInteger(chart_id,name,OBJPROP_YSIZE,sy); ObjectSetInteger(chart_id,name,OBJPROP_XDISTANCE,px); ObjectSetInteger(chart_id,name,OBJPROP_YDISTANCE,py); ObjectSetInteger(chart_id,name,OBJPROP_COLOR,txt_color); ObjectSetInteger(chart_id,name,OBJPROP_SELECTABLE,selectable); ObjectSetInteger(chart_id,name,OBJPROP_BACK,back); if(with_rectangle) ObjectSetInteger(chart_id,name,OBJPROP_BORDER_TYPE,border_type); if(with_rectangle) ObjectSetInteger(chart_id,name,OBJPROP_READONLY,true); if(with_rectangle) ObjectSetInteger(chart_id,name,OBJPROP_BGCOLOR,back_col); if(with_rectangle) ObjectSetInteger(chart_id,name,OBJPROP_BORDER_COLOR,border_col); } //CREATE BTN OBJECT ENDS HERE //Period to Timeframe ENUM_TIMEFRAMES PeriodToTF(int period) { if(period==1) return(PERIOD_M1); if(period==5) return(PERIOD_M5); if(period==15) return(PERIOD_M15); if(period==30) return(PERIOD_M30); if(period==60) return(PERIOD_H1); if(period==240) return(PERIOD_H4); if(period==1440) return(PERIOD_D1); if(period==10080) return(PERIOD_W1); if(period==43200) return(PERIOD_MN1); return(PERIOD_CURRENT); } int TFToPeriod(ENUM_TIMEFRAMES tf) { if(tf==PERIOD_CURRENT) return(Period()); if(tf==PERIOD_M1) return(1); if(tf==PERIOD_M5) return(5); if(tf==PERIOD_M15) return(15); if(tf==PERIOD_M30) return(30); if(tf==PERIOD_H1) return(60); if(tf==PERIOD_H4) return(240); if(tf==PERIOD_D1) return(1440); if(tf==PERIOD_W1) return(10080); if(tf==PERIOD_MN1) return(43200); return(Period()); } //Timeframe To String string TFtoString(ENUM_TIMEFRAMES TF) { string returnio=""; if(TF==PERIOD_M1) returnio="M1"; if(TF==PERIOD_M5) returnio="M5"; if(TF==PERIOD_M15) returnio="M15"; if(TF==PERIOD_M30) returnio="M30"; if(TF==PERIOD_H1) returnio="H1"; if(TF==PERIOD_H4) returnio="H4"; if(TF==PERIOD_D1) returnio="D1"; if(TF==PERIOD_W1) returnio="W1"; if(TF==PERIOD_MN1) returnio="MN1"; return(returnio); }
I don't know what you want to do.
I had hoped you would fiddle with it until it worked but some elements are displaced.
Thanks for your help Marco.
What I want to do is actually something very simple. The script must create two labels, one stating the current local hour, this works fine at the moment.
The problem is with the second label that should show my input, in my case some abbreviation. I saw this example: https://docs.mql4.com/basis/variables/inputvariables
I want the script to show me the input possibilities, the abbreviation, like the third screen in the example shows the days of the week.
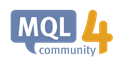
- docs.mql4.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Good day everyone,
I'm trying to make a simple script that places a label on the chart with two pieces of text, that are defined by external input at the start of the script.
The script makes the label, but when I add the external input, based on the example found here: https://docs.mql4.com/basis/variables/inputvariables,
I get the error message : " 'input' - unexpected token" so my understanding of how this should be done is not right.
Can someone please tell me how I should change the code in order to work properly?
Robert