Please show your code if you need coding help.
Thanks, updated post :)
The code seems logically correct.
You have to debug it, print the values, check the logs. And provide a code that compiles if you need more help.
It compiled, I printed the values and checked the logs - there are no issues (I'm running it on a MT5 VPS).
It still only opens one order instead of multiple (even though the entry conditions are satisfied).
This logic is somewhat confusing, and is what programmers call "spaghetti" code.
In programming, a function should do only one thing well, and every function should strive to be "pure", meaning you get a consistent output for every input. In your case, your "OpenShortTrade" is not only trying to place trades, but it's also attempting to limit trading based on some global dependencies, not good. If you trace you problem in the debugger I'd bet you'll find the issue in this function's dependencies.
This logic is somewhat confusing, and is what programmers call "spaghetti" code.
In programming, a function should do only one thing well, and every function should strive to be "pure", meaning you get a consistent output for every input. In your case, your "OpenShortTrade" is not only trying to place trades, but it's also attempting to limit trading based on some global dependencies, not good. If you trace you problem in the debugger I'd bet you'll find the issue in this function's dependencies.
I condensed what was necessary into a few lines of code, hence the output you're seeing - if 10 lines of logic is confusing to you, I'm sorry... MQL forum full of problem solvers, as usual. Issue is fixed anyway (Bars function is not reliable, at all).
I condensed what was necessary into a few lines of code, hence the output you're seeing - if 10 lines of logic is confusing to you, I'm sorry... MQL forum full of problem solvers, as usual. Issue is fixed anyway (Bars function is not reliable, at all).
So then I was right. And there's no need for the sarcasm... I was trying to help you develop your skills.
- The snippet uses unnecessary global variables which is poor form.
- Of course Bars is not reliable -- because you called it from OnInit. All functions that access timeseries data are unreliable from OnInit.
- Logic is happening in the wrong places. You don't see the number of bars checked against some global non-const in the OrderSend funcs... so don't do it in your own code. It will cause you many problems down the road once your codebase grows. This is the snippet refactored. I'm not saying it's "perfect", but this is generally how programs should flow.
#include <trade/trade.mqh> CTrade g_trade; int OnInit() { g_trade.SetExpertMagicNumber(MAGIC); } void OnTick() { static datetime last_trade_bar = 0; datetime this_bar = (datetime)SeriesInfoInteger( _Symbol, _Period, SERIES_LASTBAR_DATE ); if(this_bar != last_trade_bar && market_short(0.01)) last_trade_bar = this_bar; } bool market_short(double volume) { bool res = g_trade.Sell(volume); g_trade.PrintResult(); return res; }
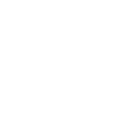
- www.learncpp.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi all,
I have been running EAs that open / close multiple trades during specific sessions. I use the CTrade class, but it occurred to me that lately only one position is being opened, instead of several. This seems to have worked before.
Could someone please alleviate me of my troubles, and post their code for opening / closing several positions? My accounts are hedge accounts, so I should be able to have several 'positions' open as far as I know.
I use the Bars() function to ensure that I only open max one trade per bar, and the code snippet to open short trades is as follows:
This code will only open up one short position though, even if called several times on different bars. Any ideas?
Best regards,
X