I need to create two classes that has child & parent relationship
parent class can create their child within constructor and set child parent to itself.
This can be possible with MQL4?
ps. Sorry, my English is not good.
Hi @Pisit Wongmaungsan - I'm fairly new to MQL4 OOP programming so, please bear that in mind. Without commenting on the efficacy of your overall solution, from a higher level language, like C#, you can obviously pass "this" in the manner you have specified.
In your Child class, you are using a pointer of type Parent. I think you would need to do the following to get what you want:
child.setParent(GetPointer(this));
Take a look at https://docs.mql4.com/common/getpointer
Hope this helps.
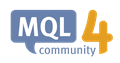
- docs.mql4.com
child.setParent(GetPointer(this));
void setParent(Parent &p) { parent = GetPointer(p); }; : child.setParent(this);And it prevents passing a NULL pointer to the child.
GetPointer(this)
That what I'm looking for. it solves my problem. Thank you so much.
That what I'm looking for. it solves my problem. Thank you so much.
Glad to help :)
That what I'm looking for. it solves my problem. Thank you so much.
You can also use
&this
I also use this pattern a lot, but pass the pointer through the constructor instead.
class Parent; class Child { Parent *m_parent; public: Child(Parent *p) { m_parent = p; } }; class Parent { Child m_child; public: Parent():m_child(&this){} };
You can also use
I also use this pattern a lot, but pass the pointer through the constructor instead.
wow. I have never seen this technique before.
Thanks for more info & suggestion.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I need to create two classes that has child & parent relationship
parent class can create their child within constructor and set child parent to itself.
This can be possible with MQL4?
ps. Sorry, my English is not good.