Hi Jon,
First things first, since you're working with MT4 you have to specify the compiler that you want to use. You always want to use the new compiler, so always begin your MQL4 projects with
#property strict
https://docs.mql4.com/mql4changes#compiler_difference
Next, there seem to be a lot of room for improvement with your opening logic. Let's see how we can improve it..
if(Open[1]<ma && Close[1]>ma || Open[0]<ma && Close[0]>ma && Close[1]-Open[1] <= 0.0030) ...
if((Open[1]<ma && Close[1]>ma || Open[0]<ma && Close[0]>ma) && fabs(Close[1]-Open[1]) <= 300*_Point) ...
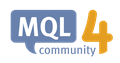
- docs.mql4.com
- Never mix ands and ors together. Always parenthese
if( Open[1]<ma && Close[1]>ma || Open[0]<ma && Close[0]>ma && Close[1]-Open[1] <= 0.0030) ambiguous if( (Open[1]<ma && Close[1]>ma) || (Open[0]<ma && Close[0]>ma && Close[1]-Open[1] <= 0.0030)) did you mean this? if( Open[1]<ma &&(Close[1]>ma || Open[0]<ma)&& Close[0]>ma && Close[1]-Open[1] <= 0.0030) or this? if(((Open[1]<ma && Close[1]>ma) || (Open[0]<ma)&& Close[0]>ma))&& Close[1]-Open[1] <= 0.0030) or this?
- Write self-documenting code
bool crossUp1 = Open[1]<ma && Close[1]>ma; bool crossUp0 = Open[0]<ma && Close[0]>ma; bool small1 = Close[1]-Open[1] <= 0.0030; if( (crossUp1 || crossUp0) && small1)
- Don't hard code constants. Code breaks on JPY pairs. If you want 30 pips compute what a pip is and use it.
How to manage JPY pairs with parameters? - MQL4 and MetaTrader 4 - MQL4 programming forum
Hi Jon,
First things first, since you're working with MT4 you have to specify the compiler that you want to use. You always want to use the new compiler, so always begin your MQL4 projects with
https://docs.mql4.com/mql4changes#compiler_difference
Next, there seem to be a lot of room for improvement with your opening logic. Let's see how we can improve it..
- Never mix ands and ors together. Always parenthese
- Write self-documenting code
- Don't hard code constants. Code breaks on JPY pairs. If you want 30 pips compute what a pip is and use it.
How to manage JPY pairs with parameters? - MQL4 and MetaTrader 4 - MQL4 programming forum
Thank you both for replying so quickly! I will try to implement these corrections/improvements next chance I get and I will update.
nicholishen: I will read up on the compiler and try your code to see if it gives me the desired result, I'll keep you updated.
whroeder1:
1. Like I said, I'm a total noob at mql4, so I'm not even entirely sure what the results of those differences in coding are. I will test them all and let you know.
2. I'm definitely working my way up to this. I haven't quite learned it yet but it is definitely something I will do.
3. I will try to keep from doing this. At the moment, I'm not too concerned with it only because this is intended for non-JPY pairs.
Again thank you both for your input and assistance!
Hi whroeder1 and nicholishen
I test all the improvements and corrections you provided. Up to now, moving around the parenthesis to correctly group the code has resolved the problem with the EA taking trades after 30+pip bodies. Thank you guys so much for the suggestions! I'm going to keep improving it, as I've come across some other issues, but I'm going to open another topic for it because it is not related to this issue.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi everyone..total noob here! You've been warned. I just want to say ahead of time that I did run a search on the forum and couldn't find what I was looking for, most likely because of the amount of information that is one here (A LOT!).
With that said, what I am attempting to do is create, very primitively, an EA that opens a trade when price closes above/below the 34EMA, but I want it to check to make sure that the previously closed candle body (open to close) did not change by more than 30 pips. There are other check I want to add for when to close, but I'll leave it at this for now. Any help would be appreciated!! Code included. Yes I did start this with the sample MA EA that came with MT4 and I don't know what a lot of it means, but I do know other forms of coding/programming so I catch on pretty quick.
Code: