I just want for the EA to not execute anything when a certain condition is met (expression1)
To execute nothing when expression1 is true:
if (expression1) { // NOOP } else { operator2 . . . }
Of course, you could just rewrite it:
if (! expression1)
{
operator2 . . .
}
To execute nothing when expression1 is true:
Of course, you could just rewrite it:
- If you just want to stop a loop and "get out", then use the "break" operator.
- If you just want to skip one iteration of the loop and advance to the next one, then use the "continue" operator.
However, if you properly structure your code, you will probably never have to use either of those two operators. So, first consider the logic of your code in detail, and make sure you are doing it correctly.
For example, changing your code into this:
for( int i = OrdersTotal() - 1; i >= 0; i-- ) // It is advisable to count down { if( OrderSelect( i, SELECT_BY_POS, MODE_TRADES ) ) { if( !expression1 ) { // Do Something } } else Print( "Error Selecting Order: ", GetLastError() ); }
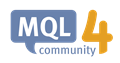
- docs.mql4.com
- If you just want to stop a loop and "get out", then use the "break" operator.
- If you just want to skip one iteration of the loop and advance to the next one, then use the "continue" operator.
However, if you properly structure your code, you will probably never have to use either of those two operators. So, first consider the logic of your code in detail, and make sure you are doing it correctly.
For example, changing your code into this:
Thank you Fernando, I should better explain the problem, so this for loop is made to put a pending order with a market order previously placed. The condition is if a market order is opened then a pending order should be opened that's it.. but the problem here is that my pending order is opened infinitely more than one time whereas I need one single pending order.
So how to avoid for PO to be opened more than one time.
Logically I thought this could work but unfortunately it repeats infinitely the PO.
for( int i = OrdersTotal() - 1; i >= 0; i-- ) { if( OrderSelect( i, SELECT_BY_POS, MODE_TRADES ) ) { if( !(OrderSymbol() == Symbol() && OrderMagicNumber() == Magic && OrderType() > 1) ) { // PO function. } } else Print( "Error Selecting Order: ", GetLastError() ); }
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 and MetaTrader 4 - MQL4 programming forum

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I just want for the EA to not execute anything when a certain condition is met (expression1)