Hello everyone,
I'm experiencing problems with the my first part of the code isn't interacting with the second part. How could it be possible to change the String to a OrderSend?
If you're placing an order on a symbol that is different from the chart's symbol, you can't use the pre-defined variables of Bid, Ask, Point or Digits, or the current symbol's stoplevel. You need to retrieve them using MarketInfo or SymbolInfoDouble.
e.g.
string symbol = "EURUSD"; double ask = SymbolInfoDouble(symbol,SYMBOL_ASK), bid = SymbolInfoDouble(symbol,SYMBOL_BID), pnt = SymbolInfoDouble(symbol,SYMBOL_POINT); int digits = (int)SymbolInfoInteger(symbol,SYMBOL_DIGITS);
When you do the OrderSend():
int ticket = OrderSend(symbol, OP_BUY, lots, ask,...
Also, although it is very unlikely to get a ticket number of 0, it is arguably possible. An OrderSend() failure would return -1:
//if(ticket<1) if(ticket<0) { Print("Debug",GetLastError()); }
My wishfully idea to do something like this:
input ENUM_NAME_SYMBOL NEW_SELECTION =MODE_TRADES;
NEW_SELECTION = MODE_TRADES;
Pair_1,
Pair_2,
Pair_3...
My wishfully idea to do something like this:
NEW_SELECTION = MODE_TRADES;
Pair_1,
Pair_2,
Pair_3...
I'm sorry, I'm not 100% following.
MODE_TRADES is actually a term used for a pool in OrderSelect. You'll probably want to choose another name.
Do you mean something like this?
Dropdown list in inputs:
enum symbols { EURUSD,USDJPY,AUDCAD }; input symbols symbol = EURUSD;
You'll need to convert that back to a string (enumerations aren't strings):
string sym = EnumToString(symbol); // you'll also need to deal with broker suffix / prefix
Then you can place your orders:
double ask = SymbolInfoDouble(sym,SYMBOL_ASK), bid = SymbolInfoDouble(sym,SYMBOL_BID), pnt = SymbolInfoDouble(sym,SYMBOL_POINT); int digits = (int)SymbolInfoInteger(sym,SYMBOL_DIGITS); int ticket = OrderSend(sym,OP_BUY,lots,ask,...
Or have I missed your point entirely?
I'm sorry, I'm not 100% following.
MODE_TRADES is actually a term used for a pool in OrderSelect. You'll probably want to choose another name.
Do you mean something like this?
Dropdown list in inputs:
You'll need to convert that back to a string (enumerations aren't strings):
Then you can place your orders:
Or have I missed your point entirely?
Thank you, honest_knave
That Enum has been doing my head in for weeks.
- You can do this if you really want to but remember the admonition:honest_knave: If you're placing an order on a symbol that is different from the chart's symbol, you can't use the pre-defined variables of Bid, Ask, Point or Digits, or the current symbol's stoplevel. You need to retrieve them using MarketInfo or SymbolInfoDouble.
- This is why I recommendDo not trade multiple currencies in one EA
- You can't use any predefined variables,
can't use the
tester, must poll (not OnTick,) and usually other problems, e.g A problem with iBarShift - MQL4 and MetaTrader 4 - MQL4
programming forum - Page 2
- Code it to trade the chart pair only. Look at the others if you must. (Don't assume that Time[i] == iTime(otherPair,tf, i) always use iBarShift.)
- Then put it on other charts to trade the other pairs. Done.
- You can't use any predefined variables,
can't use the
tester, must poll (not OnTick,) and usually other problems, e.g A problem with iBarShift - MQL4 and MetaTrader 4 - MQL4
programming forum - Page 2
Hello again,
I am just experimenting writing EA's at the moment.
To whroeder1, I don't plan on trading any more than three different currencies on a single EA.
To honest_knave, I don't know what you mean by "you'll also need to deal with broker suffix/prefix"
I have tried this code below.
void StartBuyOrders() { string sym = EnumToString(symbol); // you'll also need to deal with broker suffix / prefix double ask = SymbolInfoDouble(sym,SYMBOL_ASK), bid = SymbolInfoDouble(sym,SYMBOL_BID), pnt = SymbolInfoDouble(sym,SYMBOL_POINT); int digits = (int)SymbolInfoInteger(sym,SYMBOL_DIGITS); int ticket=OrderSend(sym,OP_BUY,Amount,MODE_ASK,4,MODE_STOPLEVEL,MODE_SPREAD ,"EA Order",0,0,clrBlue); if(ticket<1) { Print("Debug",GetLastError()); } else Print("Order successfully"); }
The Terminal Log reads:
order buy 0.03 USDJPY opening at market sl: 14.000 tp: 13.000 failed [Market is closed]
The SL and TP have me a bit confused atm.
To honest_knave, I don't know what you mean by "you'll also need to deal with broker suffix/prefix"
Some brokers use a suffix on their symbols. For example, EURUSD might be EURUSDm or EURUSD-sb
In these situations, trying to open an order on "EURUSD" is going to fail. You would need to open the order using exact name of the symbol for that particular broker.
The SL and TP have me a bit confused atm.
You have to set your TP and SL as a price, not a distance. So if you enter short at 1.10000 and you want a 10 pip SL you would need to set 1.10100 (not 10).
You also should remember that on most forex brokers these days, 10 pips = 100 points. MQL4 uses points not pips.
Take a look at the OrderSend documentation which gives an example that you can then modify.
The Terminal Log reads:
order buy 0.03 USDJPY opening at market sl: 14.000 tp: 13.000 failed [Market is closed]
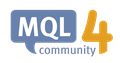
- docs.mql4.com
Thank you, honest_knave
I am still learning how to trade successfully using MQL4.
Does MQL5 use both pips & points?
honest_knave: Some brokers use a suffix on their symbols. For example, EURUSD might be EURUSDm or EURUSD-sb
|
|
|
Thank you, whroeder1,
Just a bit advanced for me.
for the attached file. Would you be able to comment on the "class" ?
I'm not sure this is thread related...

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello everyone,
I'm experiencing problems with the my first part of the code isn't interacting with the second part. How could it be possible to change the String to a OrderSend?