Use a struct.
struct data { double d; string s; }; data test[3] = { {1.11, "test1"}, {2.22, "test2"}, {3.33,"test3"} };
Papillon:
Use a struct.
struct data { double d; string s; }; data test[3] = { {1.11, "test1"}, {2.22, "test2"}, {3.33,"test3"} }; for(int i=0;i<3;i++) { Print("TestValue: ", test[i] ); } //Result: Compiler Error - 'test' - objects are passed by reference only
Thanks for the answer.
But how to call the values?
Sorry, I am newbie on MQL4
wieb:
Thanks for the answer.
But how to call the values?
Sorry, I am newbie on MQL4
struct data { double d; string s; }; data test[3] = { {1.11, "test1"}, {2.22, "test2"}, {3.33,"test3"} }; for(int i=0;i<3;i++) { printf("TestValue: %f %s", test[i].d, test[i].s ); }https://www.mql5.com/en/docs/basis/types/classes
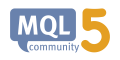
Documentation on MQL5: Language Basics / Data Types / Structures, Classes and Interfaces
- www.mql5.com
Language Basics / Data Types / Structures, Classes and Interfaces - Reference on algorithmic/automated trading language for MetaTrader 5
Thanks a lot for all the help
struct data { double d; string s; }; void test(data& testx[]) { ArrayResize(testx,3,3); for(int i=0;i<3;i++) { testx[i].d = 1.11+i; testx[i].s = "TEST" + (string)i; } } void OnStart() { double test[3]; for(int i=0;i<3;i++) { printf("TestValue: %f %s", test[i].d, test[i].s ); } } //Results - Error - 'd' - struct or class type expected - 's' - struct or class type expected
How to solve this?
Thanks.
wieb:
How to solve this?
Thanks.
struct data { double d; string s; }; void test(data& testx[]) { ArrayResize(testx,3,3); for(int i=0;i<3;i++) { testx[i].d = 1.11+i; testx[i].s = "TEST" + (string)i; } } void OnStart() { data test[3]; for(int i=0;i<3;i++) { printf("TestValue: %f %s", test[i].d, test[i].s ); } } //Finally - no compiler errors - but wrong results //Results: //TestValue: 0.000000 (null) //TestValue: 0.000000 (null) //TestValue: 0.000000 (null)
How to fill array in test function (not OnStart function) and the result to be like this:
TestValue: 1.110000 test1
TestValue: 2.220000 test2
TestValue: 3.330000 test3
Thanks.
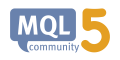
Documentation on MQL5: Array Functions / ArrayFill
- www.mql5.com
Array Functions / ArrayFill - Reference on algorithmic/automated trading language for MetaTrader 5
wieb:
How to fill array in test function (not OnStart function) and the result to be like this:
TestValue: 1.110000 test1
TestValue: 2.220000 test2
TestValue: 3.330000 test3
Thanks.
You never call your function.
struct data { double d; string s; }; void test(data& testx[]) { ArrayResize(testx,3,3); for(int i=0;i<3;i++) { testx[i].d = 1.11+i; testx[i].s = "TEST" + (string)i; } } void OnStart() { data test[3]; test(test); for(int i=0;i<3;i++) { printf("TestValue: %f %s", test[i].d, test[i].s ); } }
honest_knave:
You never call your function.
Thank you very much for all the help, now it works as expected.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
How to make result like this:
1.11, test1
2.22, test2
3.33, test3
Thanks for the help.