//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime& time[],
const double& open[],
const double& high[],
const double& low[],
const double& close[],
const long& tick_volume[],
const long& volume[],
const int& spread[])
{
int limit = rates_total - prev_calculated;
//--- counting from 0 to rates_total
ArraySetAsSeries(Buffer1, true);
ArraySetAsSeries(Buffer2, true);
//--- initial zero
if(prev_calculated < 1)
{
ArrayInitialize(Buffer1, 0);
ArrayInitialize(Buffer2, 0);
}
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
int DoAlerts()
{...}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int start()
{
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime& time[],
const double& open[],
const double& high[],
const double& low[],
const double& close[],
const long& tick_volume[],
const long& volume[],
const int& spread[])
{
int limit = rates_total - prev_calculated;
//--- counting from 0 to rates_total
ArraySetAsSeries(Buffer1, true);
ArraySetAsSeries(Buffer2, true);
//--- initial zero
if(prev_calculated < 1)
{
ArrayInitialize(Buffer1, 0);
ArrayInitialize(Buffer2, 0);
}
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
int DoAlerts()
{...}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int start()
{
- Post code that compiles. No closing brace on OnCalculate
- OnCalculate or start, not both.
- Why are you setting your buffers to zero? A) They are pre-loaded with EMPTY_VALUE since you didn't change the default. Thus a line will be drawn from price=zero to what you set a buffer to.
- Do your lookbacks
correctly.
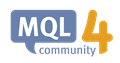
Other constants - Named Constants - Standard Constants, Enumerations and Structures - MQL4 Reference
- docs.mql4.com
Other constants - Named Constants - Standard Constants, Enumerations and Structures - MQL4 Reference

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
A little help, please?
#property description ""
#include <stdlib.mqh>
#include <stderror.mqh>
//--- indicator settings
#property indicator_chart_window
#property indicator_buffers 2
#property indicator_style1 STYLE_DASHDOTDOT
#property indicator_width1 2
#property indicator_color1 Red
#property indicator_label1 "H3"
#property indicator_style2 STYLE_DASHDOTDOT
#property indicator_width2 2
#property indicator_color2 Lime
#property indicator_label2 "L3"
//---- input parameters
extern bool Alerts = false;
extern double GMTshiftSun = 0; //sun into mon cams use this corrected value for fri data
extern double GMTshift = 1;
extern bool Pivot = true;
extern bool CamTargets = true;
extern bool ListCamTargets = true;
extern color PivotColor = LimeGreen;
extern color PivotFontColor = White;
extern int PivotFontSize = 8;
extern int PivotWidth = 1;
extern int PipDistance = 20;
extern color CamFontColor = Gray;
extern int CamFontSize = 10;
extern bool Fibs = true;
extern color FibColorRes = SeaGreen;
extern color FibColorSup = Brown;
extern color FibFontColor = Gray;
extern int FibFontSize = 8;
extern bool DisplayFibLevels_1_3 = false;
extern double FibLevel1 = 0.236;
extern double FibLevel2 = 0.382;
extern double FibLevel3 = 0.50;
extern bool DisplayFibLevels_4_6 = true;
extern double FibLevel4 = 0.618;
extern double FibLevel5 = 0.764;
extern double FibLevel6 = 0.99;
extern bool DisplayFibLevels_7_16 = true;
extern double FibLevel7 = 1.27;
extern double FibLevel8 = 1.618;
extern double FibLevel9 = 1.99;
extern double FibLevel10 = 2.236;
extern double FibLevel11 = 2.618;
extern double FibLevel12 = 2.99;
extern double FibLevel13 = 3.236;
extern double FibLevel14 = 3.618;
extern double FibLevel15 = 3.99;
extern double FibLevel16 = 4.236;
extern bool StandardPivots = true;
extern bool ListStandardPivots = true;
extern color StandardFontColor = Gray;
extern int StandardFontSize = 8;
extern color SupportColor = Brown;
extern color ResistanceColor = SeaGreen;
extern bool MidPivots = false;
extern color MidPivotColor = White;
extern int MidFontSize = 8;
bool BufferOK = true;
double P, H3, H4, H5;
double L3, L4, L5;
double LastHigh,LastLow,x;
bool firstL3=true;
bool firstH3=true;
double D1= 0.091667;
double D2= 0.183333;
double D3= 0.2750;
double D4= 0.55;
// Fib variables
double yesterday_high=0;
double yesterday_low=0;
double yesterday_close=0;
double p=0;
double r1=0,r2=0,r3=0,r4=0,r5=0,r6=0,r7=0,r8=0,r9=0,r10=0,r11=0,r12=0,r13=0,r14=0,r15=0,r16=0;
double s1=0,s2=0,s3=0,s4=0,s5=0,s6=0,s7=0,s8=0,s9=0,s10=0,s11=0,s12=0,s13=0,s14=0,s15=0,s16=0;
double R;
//--- indicator buffers
double Buffer1[];
double Buffer2[];
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
IndicatorBuffers(2);
SetIndexBuffer(0,Buffer1);
SetIndexStyle (0,DRAW_LINE,STYLE_SOLID,2);
SetIndexLabel (0, "H3 SHORT");
SetIndexBuffer(1,Buffer2);
SetIndexStyle (1,DRAW_LINE,STYLE_DOT,1);
SetIndexLabel(1,"L3 LONG" );
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime& time[],
const double& open[],
const double& high[],
const double& low[],
const double& close[],
const long& tick_volume[],
const long& volume[],
const int& spread[])
{
int limit = rates_total - prev_calculated;
//--- counting from 0 to rates_total
ArraySetAsSeries(Buffer1, true);
ArraySetAsSeries(Buffer2, true);
//--- initial zero
if(prev_calculated < 1)
{
ArrayInitialize(Buffer1, 0);
ArrayInitialize(Buffer2, 0);
}
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
int DoAlerts()
{
double DifAboveL3,PipsLimit;
double DifBelowH3;
DifBelowH3 = H3 - Close[0];
DifAboveL3 = Close[0] - L3;
PipsLimit = PipDistance*Point;
if (DifBelowH3 > PipsLimit) firstH3 = true;
if (DifBelowH3 <= PipsLimit && DifBelowH3 > 0)
{
if (firstH3)
{
Alert("Below Cam H3 Line by ",DifBelowH3, " for ", Symbol(),"-",Period());
PlaySound("alert.wav");
firstH3=false;
}
}
if (DifAboveL3 > PipsLimit) firstL3 = true;
if (DifAboveL3 <= PipsLimit && DifAboveL3 > 0)
{
if (firstL3)
{
Alert("Above Cam L3 Line by ",DifAboveL3," for ", Symbol(),"-",Period());
Sleep(2000);
PlaySound("timeout.wav");
firstL3=false;
}
}
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int start()
{
int counted_bars=IndicatorCounted();
//---- TODO: add your code here
double day_high=0;
double day_low=0;
double yesterday_open=0;
double today_open=0;
double Q=0,S=0,R=0,M2=0,M3=0,S1=0,R1=0,M1=0,M4=0,S2=0,R2=0,M0=0,M5=0,S3=0,R3=0,nQ=0,nD=0,D=0,R4=0,S4=0,R5=0,S5=0;
double shift_corrected;
int cnt=720;
double cur_day=0;
double prev_day=0;
double rates_d1[2][6];
//---- exit if period is greater than daily charts
if(Period() > 1440)
{
Print("Error - Chart period is greater than 1 day.");
return(-1); // then exit
}
//---- Get new daily prices & calculate pivots
if (DayOfWeek()==1) shift_corrected=GMTshiftSun;
else if ((DayOfWeek()==2) && (Minute() >= 5)) shift_corrected=GMTshift;
//else if ((DayOfWeek()==2) && (Hour() > GMTshift)) shift_corrected=GMTshift;
else shift_corrected=GMTshift;
while (cnt!= 0)
{
cur_day = TimeDay(Time[cnt]- (shift_corrected*3600));
if (prev_day != cur_day)
{
yesterday_close = Close[cnt+1];
today_open = Open[cnt];
yesterday_high = day_high;
yesterday_low = day_low;
day_high = High[cnt];
day_low = Low[cnt];
prev_day = cur_day;
}
if (High[cnt]>day_high)
{
day_high = High[cnt];
}
if (Low[cnt]<day_low)
{
day_low = Low[cnt];
}
cnt--;
}
D = (day_high - day_low);
Q = (yesterday_high - yesterday_low);
//------ Pivot Points ------
P = (yesterday_high + yesterday_low + yesterday_close)/3;//Pivot
//---- To display all 8 Camarilla pivots remove comment symbols below and
// add the appropriate object functions below
H5 = (yesterday_high/yesterday_low)*yesterday_close;
H4 = ((yesterday_high - yesterday_low)* D4) + yesterday_close;
H3 = ((yesterday_high - yesterday_low)* D3) + yesterday_close;
//H2 = ((yesterday_high - yesterday_low) * D2) + yesterday_close;
//H1 = ((yesterday_high - yesterday_low) * D1) + yesterday_close;
//L1 = yesterday_close - ((yesterday_high - yesterday_low)*(D1));
//L2 = yesterday_close - ((yesterday_high - yesterday_low)*(D2));
L3 = yesterday_close - ((yesterday_high - yesterday_low)*(D3));
L4 = yesterday_close - ((yesterday_high - yesterday_low)*(D4));
L5 = yesterday_close - (H5 - yesterday_close);
if (Fibs)
{
R = yesterday_high - yesterday_low;//range
p = (yesterday_high + yesterday_low + yesterday_close)/3;// Standard Pivot
if (DisplayFibLevels_1_3){
r1 = p + (R * FibLevel1);
r2 = p + (R * FibLevel2);
r3 = p + (R * FibLevel3);
s1 = p - (R * FibLevel1);
s2 = p - (R * FibLevel2);
s3 = p - (R * FibLevel3);}
if (DisplayFibLevels_4_6){
r4 = p + (R * FibLevel4);
r5 = p + (R * FibLevel5);
r6 = p + (R * FibLevel6);
s4 = p - (R * FibLevel4);
s5 = p - (R * FibLevel5);
s6 = p - (R * FibLevel6);}
if (DisplayFibLevels_7_16){
r7 = p + (R * FibLevel7);
r8 = p + (R * FibLevel8);
r9 = p + (R * FibLevel9);
r10 = p + (R * FibLevel10);
r11 = p + (R * FibLevel11);
r12 = p + (R * FibLevel12);
r13 = p + (R * FibLevel13);
r14 = p + (R * FibLevel14);
r15 = p + (R * FibLevel15);
r16 = p + (R * FibLevel16);
s7 = p - (R * FibLevel7);
s8 = p - (R * FibLevel8);
s9 = p - (R * FibLevel9);
s10 = p - (R * FibLevel10);
s11 = p - (R * FibLevel11);
s12 = p - (R * FibLevel12);
s13 = p - (R * FibLevel13);
s14 = p - (R * FibLevel14);
s15 = p - (R * FibLevel15);
s16 = p - (R * FibLevel16);}
}
if (StandardPivots)
{
R1 = (2*P)-yesterday_low;
S1 = (2*P)-yesterday_high;
R2 = P-S1+R1;
S2 = P-R1+S1;
R3 = (2*P)+(yesterday_high-(2*yesterday_low));
S3 = (2*P)-((2* yesterday_high)-yesterday_low);
R4 = (3*P)+(yesterday_high-(3*yesterday_low));
S4 = (3*P)-((3* yesterday_high)-yesterday_low);
R5 = (4*P)+(yesterday_high-(4*yesterday_low));
S5 = (4*P)-((4* yesterday_high)-yesterday_low);
}
if (MidPivots)
{
M0 = (S2+S3)/2;
M1 = (S1+S2)/2;
M2 = (P+S1)/2;
M3 = (P+R1)/2;
M4 = (R1+R2)/2;
M5 = (R2+R3)/2;
}
for(int i=0;i<iBars(Symbol(),Period());i++)
{
//--- Daily
if(BufferOK==true)
{
Buffer1[i]=H3;
Buffer2[i]=L3;
}
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
return(rates_total);
}
//+------------------------------------------------------------------+