- Indicators with alerts/signal
- Russian guides for the RTS
- Moving average EA
Generally, this is a beginners problem. You do-not want to use static-variables or global-variables for that matter. You already have the logic for this and that should be sufficient. You need Four MA Values and not 2.
double Fast_Ma_Current=iCustom(Symbol(),0,"Whatever_Name",Whatever_Buffer,0);//Where Shift 0=Current.
double Fast_Ma_Previous=iCustom(Symbol(),0,"Whatever_Name",Whatever_Buffer,1);// Shift Bar Before Current.
double Slow_Ma_Current=iCustom(Symbol(),0,"Whatever_Name",Whatever_Buffer,0);//Where Shift 0=Current.
double Slow_Ma_Previous=iCustom(Symbol(),0,"Whatever_Name",Whatever_Buffer,1);//Shift Bar Before Current.
if( Fast_Ma_Previous<Slow_Ma_Previous && Fast_Ma_Current>Slow_Ma_Current) { int Direction= 1; }//Buy_Trigger
if( Fast_Ma_Previous>Slow_Ma_Previous && Fast_Ma_Current<Slow_Ma_Current) { Direction= -1; }//Sell_Trigger
Within the above, the int Direction is a Local-Variable and will Reset to Zero when things don't cross. You could also define the Local variable before the IF.
Generally, this is a beginners problem. You do-not want to use static-variables or global-variables for that matter. You already have the logic for this and that should be sufficient. You need Four MA Values and not 2.
double Fast_Ma_Current=iCustom(Symbol(),0,"Whatever_Name",Whatever_Buffer,0);//Where Shift 0=Current.
double Fast_Ma_Previous=iCustom(Symbol(),0,"Whatever_Name",Whatever_Buffer,1);// Shift Bar Before Current.
double Slow_Ma_Current=iCustom(Symbol(),0,"Whatever_Name",Whatever_Buffer,0);//Where Shift 0=Current.
double Slow_Ma_Previous=iCustom(Symbol(),0,"Whatever_Name",Whatever_Buffer,1);//Shift Bar Before Current.
if( Fast_Ma_Previous<Slow_Ma_Previous && Fast_Ma_Current>Slow_Ma_Current) { int Direction= 1; }//Buy_Trigger
if( Fast_Ma_Previous>Slow_Ma_Previous && Fast_Ma_Current<Slow_Ma_Current) { Direction= -1; }//Sell_Trigger
Within the above, the int Direction is a Local-Variable and will Reset to Zero when things don't cross. You could also define the Local variable before the IF.
Thanks for this. I am guilty as charged- a Newbie, but I am learning thanks to you Guys!!
I will give this a go
double curDir = Fast_Ma_Current - Slow_Ma_Current, preDir = Fast_Ma_Previous - Slow_Ma_Previous; bool cross = curDir * PreDIR < 0; if (cross){ if (curDir > 0) // Buy : }
Now you are just showing off! ;-)
WHR's code, which admittedly is pretty good.
double curDir = Fast_Ma_Current - Slow_Ma_Current, preDir = Fast_Ma_Previous - Slow_Ma_Previous; bool cross = curDir * PreDIR < 0; if (cross){ if (curDir > 0) // Buy : }
And my effort to make a more efficient test (using bools rather than doubles.)
bool curRise = Fast_Ma_Current > Slow_Ma_Current; bool prevRise = Fast_Ma_Previous > Slow_Ma_Previous; bool cross = (curRise!=prevRise); if( cross ){ if( curRise ){ // Buy } }
WHR's code, which admittedly is pretty good.
And my effort to make a more efficient test (using bools rather than doubles.)
Now you are just showing off! ;-)
Dam it dabbler. Here I was basking in my glory
and you have to show me up, eliminate a FP multiply and replace 2 FP subtractions with faster comparisons. I guess I'll have to stick with my terse comments.
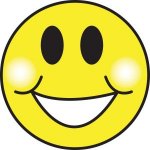
Well, if I realised I was going to start a 'Cat Fight' I would have simply said "Thank You! ;-)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use