I found a solution for anyone interested. Probably not the best way to do it but seems to work.
First another while loop to check and wait until the limit order has become an open position:
while (!PositionSelectByTicket(ticket)) { Sleep(1000); // Wait for 1 second before checking again Print("No ticket: ", PositionSelectByTicket(ticket)); }
Then I use the following code to extract the information:
HistorySelect(start,TimeTradeServer()); for(int t=0; t<HistoryDealsTotal(); t++) { ulong deal_ticket = HistoryDealGetTicket(t); if(HistoryDealGetInteger(deal_ticket,DEAL_TYPE)==DEAL_TYPE_SELL) { if(t==HistoryDealsTotal()-1) { Print(HistoryDealGetDouble(deal_ticket,DEAL_PRICE)); DebugBreak(); .....
There is no need to create pending orders in code.
-
The pending has the slight advantage, A) you are closer to the top of the queue (filled quicker), B) there's no round trip network delay (filled quicker.)
Don't worry about it unless you're scalping M1 or trading news.
-
Humans can't watch the screen 24/7, so they use pending orders; EAs can, so no need for pending orders, have it wait until the market reaches the trigger price and just open an order.
I found a solution for anyone interested. Probably not the best way to do it but seems to work.
First another while loop to check and wait until the limit order has become an open position:
Then I use the following code to extract the information:
Your solution (while..) blocks your EA. Have a look at OnTradeTransaction().
But it is tricky :(
as not always the values of MagicNumber and the IDs of the position order and/or deal are assigned to trans., req., res. and one act like send an order causes this function to 'pop up' between one and four times :(
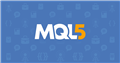
- www.mql5.com
Hi Carl, I already had a look at OnTradeTransaction as well, but it was not clear to me how to extract the needed information. As you said, it seems to be tricky...
Yes, you are right that the while loop blocks the EA but between sending the limit order and its filling nothing really needs to be done by my EA anyhow. Since, at least, in my case, I have only one position open at a time, so I would not block the checking for other positions stop losses or anything else like that. Maybe I am currently overseeing something but it'll pop up later then ;-)
Enjoy your day!
Hi Carl, I already had a look at OnTradeTransaction as well, but it was not clear to me how to extract the needed information. As you said, it seems to be tricky...
Yes, you are right that the while loop blocks the EA but between sending the limit order and its filling nothing really needs to be done by my EA anyhow. Since, at least, in my case, I have only one position open at a time, so I would not block the checking for other positions stop losses or anything else like that. Maybe I am currently overseeing something but it'll pop up later then ;-)
Enjoy your day!
The right solution is OnTradeTransaction(). You just have to learn how to use it correctly.
There are several articles about it, for example this one : https://www.mql5.com/en/articles/1111
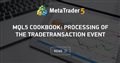
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello, I use limit orders. Since it might take some time for these orders to get filled and become an open position, I want to wait/pause code until the limit order has been filled and becomes an open position to then collect the open price and open time etc. for this position.
When I used market orders I was able to receive CT.ResultPrice() and other information and just continue with the code.
Without the while loop, my limit order is send and after a second or so it gets filled so all fine. However, when I use the while loop it gets stuck there until the strategy tester ends saying the end of the backtest is reached?
Any ideas how to handle this?