That's because the local trading environment is not update synchronously with the server. That means, as your code is executed on each tick, it can be executed again before PositionTotal() (and so all position information) is updated. Not always, it depends of the ticks and the server response to your request.
- Tick X : trade request send, server answer it's ok. But Positions are NOT UPDATED yet.
- Tick X+1 : Positions still not updated, your code send an other trade request => double trades
- The terminal receive the Positions update information.
- Tick X+2 : All is ok now, but you have a double trades.
Your code needs to deal with that.
And how can I solve that?
To clarify, I am not using a VPS or something similar.
To clarify, I am not using a VPS or something similar.
It's not related to any computer or whatever, it's how MT5 works.
With creativity. Or check for an existing solution on the forum or codebase, now you know what the problem is.
It's not related to any computer or whatever, it's how MT5 works.
Okay, thank you. I will try to find something. I found this article that could be interesting: https://www.mql5.com/es/articles/159
And also the second operation is executed exactly at the same milisecond, which is a bit suspicious. For me it is difficult to understand that the code can be executed twice and the tick can change more than once in exactly 1 milisecond.
Btw, the other problem, do you know why it is happening? Every time I open a position in the real/live account, it is closed automatically and I do not know why. I am completely lost :(
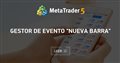
- www.mql5.com
Okay, thank you. I will try to find something. I found this article that could be interesting: https://www.mql5.com/es/articles/159
And also the second operation is executed exactly at the same milisecond, which is a bit suspicious. For me it is difficult to understand that the code can be executed twice and the tick can change more than once in exactly 1 milisecond.
Btw, the other problem, do you know why it is happening? Every time I open a position in the real/live account, it is closed automatically and I do not know why. I am completely lost :(
You will have to debug your code, sorry but I will not do it for you. If your position is closed, it's because your conditions to close triggered. Use the Debugger and/or print the needed values to understand in the log.
If somebody is curious, this is what I did:
To solve the first problem of the repeated operations, I wrote PositionsTotal() == 0 && OrdersTotal() == 0 at the beginning of the operations. I also included the CisNewBar class. I already had something very similar with (barsTotal < iBars), but anyway, I hope this is more reliable.
For the second problem, I am now going to debug it.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I leave here the code to see if you see any problem. I thought it had to do with the barsTotal < bars, but I think that's correct.