Actually I just want to understand why the author uses const here:
void CRSIAverage::SetRSIPointers(const CRSIIndividual &rsi_objects[]) { int total = ArraySize(rsi_objects); ArrayResize(rsi_indicators, total); for (int i=0; i<total; i++) rsi_indicators[i] = (CRSIIndividual*)GetPointer(rsi_objects[i]); }
It's just "good manners" and the const does not affect anything? Or is there some sence here that I don't know?
Code taken from this article.
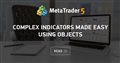
- www.mql5.com
In order to prevent changing of a parameter passed by reference, use the const modifier
Everything is clear here:
But I can change the object in this way:
That is, the const modifier protects against accidental change. But if you wish, you can change the passed object. Do I understand correctly?
Actually I just want to understand why the author uses const here:
It's just "good manners" and the const does not affect anything? Or is there some sence here that I don't know?
Code taken from this article.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
In order to prevent changing of a parameter passed by reference, use the const modifier
Everything is clear here:
But I can change the object in this way:
That is, the const modifier protects against accidental change. But if you wish, you can change the passed object. Do I understand correctly?