-
//+------------------------------------------------------------------+ // Main function to run the trading strategy void OnTick() ⋮ //+-----------+ //| Main | //+-----------+ int OnStart() ⋮
OnTick is for EAs. OnStart is for scripts. You can't have both. Program Running - MQL4 programs - MQL4 Reference
-
Use the debugger or print out your variables, including _LastError and prices and find out why. Do you really expect us to debug your code for you?
Code debugging - Developing programs - MetaEditor Help
Error Handling and Logging in MQL5 - MQL5 Articles (2015)
Tracing, Debugging and Structural Analysis of Source Code - MQL5 Articles (2011)
Introduction to MQL5: How to write simple Expert Advisor and Custom Indicator - MQL5 Articles (2010) -
while(OrdersTotal()>0)
Magic number only allows an EA to identify its trades from all others. Using OrdersTotal/OrdersHistoryTotal (MT4) or PositionsTotal (MT5), directly and/or no Magic number/symbol filtering on your OrderSelect / Position select loop means your code is incompatible with every EA (including itself on other charts and manual trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 programming forum (2013)
PositionClose is not working - MQL5 programming forum (2020)
MagicNumber: "Magic" Identifier of the Order - MQL4 Articles (2006)
Orders, Positions and Deals in MetaTrader 5 - MQL5 Articles (2011)
Limit one open buy/sell position at a time - General - MQL5 programming forum (2022)You need one Magic Number for each symbol/timeframe/strategy. Trade current timeframe, one strategy, and filter by symbol requires one MN.
Simple answer – don’t; use OnStart and OnTick in the same code. Just copy your code from OnStart into OnTick function and it should work.
Simple answer – don’t; use OnStart and OnTick in the same code. Just copy your code from OnStart into OnTick function and it should work.
Hi thank you for answer. i modified it that way.... but still i cant get the orders to close. i get an orderclose error 4051.
void OnTick() { //--- // new local variable to hold the current open time of the furthest right bar datetime rightBarTime = iTime(_Symbol,_Period,0); // check if furthest right bar has the same open time as our global varible if(rightBarTime != gBarTime) { // set the global variable to be the time of the new bar gBarTime = rightBarTime; // call out on bar function OnBar(); } } void OnBar() { double current_lot = initial_lot * MathPow(lot_multiplier, order_count); double BuyLots=0.0, SellLots=0.0, BuyProfit=0.0, SellProfit=0.0; int gBuyTicket = 0; int gSellTicket = 0; int gBuyStopTicket = 0; int gSellStopTicket = 0; if (!isBuyPositionOpen() && !isSellPositionOpen() && isPreviousCandleGreen() && order_count < max_orders) // Buy Position { gBuyTicket = OrderSend(Symbol(), OP_BUY, current_lot, Ask, 3, 0, 0, "Buy Order", 16384, 0, Green); if (gBuyTicket < 0) Print("Error opening buy order: "); else order_count++; BuyProfit += OrderProfit() + OrderCommission() + OrderSwap(); } if (!isSellPositionOpen() && !isBuyPositionOpen() && isPreviousCandleRed() && order_count < max_orders) //Sell Position { gSellTicket = OrderSend(Symbol(), OP_SELL, current_lot, Bid, 3, 0, 0, "Sell Order", 16384, 0, Red); if (gSellTicket < 0) Print("Error opening sell order: "); else order_count++; SellProfit += OrderProfit() + OrderCommission() + OrderSwap(); } if (isBuyPositionOpen() && isPreviousCandleRed() && isSecondPreviousCandleGreen() && order_count < max_orders) //SellStop Position { gSellStopTicket = OrderSend(Symbol(), OP_SELLSTOP, current_lot, Bid - (stop_loss * Point), 3, 0, 0, "SellStop Order", 16384, 0, Red); if (gSellStopTicket < 0) Print("Error opening sellstop order: "); else order_count++; SellProfit += OrderProfit() + OrderCommission() + OrderSwap(); } if (isSellPositionOpen() && isPreviousCandleGreen() && isSecondPreviousCandleRed() && order_count < max_orders) //BuyStop Position { gBuyStopTicket = OrderSend(Symbol(), OP_BUYSTOP, current_lot, Ask + (stop_loss * Point), 3, 0, 0, "BuyStop Order", 16384, 0, Green); if (gBuyStopTicket < 0) Print("Error opening buystop order: "); else order_count++; BuyProfit += OrderProfit() + OrderCommission() + OrderSwap(); } if (BuyProfit+SellProfit >= ProfitTarget) if (!DoNotCloseOrders) { closeAllPositions(gBuyTicket && gSellTicket && gBuyStopTicket && gSellStopTicket); } string sprofit=AccountCurrency()+" total Profit (current: "+DoubleToStr(BuyProfit+SellProfit,2)+" "+AccountCurrency()+")"; Comment(" Comments Last Update 13-12-2013", NL,//Comments Last Update 12-12-2006 10:00pm", NL, " Close Mode: at ",DoubleToStr(ProfitTarget,2)," "+sprofit, NL, " Buys ", OrdersBUY, NL, " BuyLots ", BuyLots, NL, " Sells ", OrdersSELL, NL, " SellLots ", DoubleToStr(SellLots,2), NL, NL, " Balance ", DoubleToStr(AccountBalance(),2)," ",AccountCurrency(), NL, " Equity ", DoubleToStr(AccountEquity(),2)," ",AccountCurrency(), NL, " Margin ", DoubleToStr(AccountMargin(),2)," ",AccountCurrency(), NL, " Free Margin ", DoubleToStr(AccountFreeMargin(),2)," ",AccountCurrency(), NL, " Current Time is ",TimeHour(CurTime()),":",TimeMinute(CurTime()),".",TimeSeconds(CurTime())); } // Function to close all open positions void closeAllPositions(int pTicket) { while(IsTradeContextBusy()) { Sleep(50); } if (OrderSelect(pTicket, SELECT_BY_POS, MODE_TRADES)) { Alert("Order Exists: ", pTicket); double lots = OrderLots(); double price = 0; if(OrderType() == OP_BUY) price = Bid; else if(OrderType() == OP_SELL) price = Ask; else if(OrderType() == OP_SELLSTOP) price = Ask; else if(OrderType() == OP_BUYSTOP) price = Bid; bool closed = OrderClose(pTicket, lots, price, 15, Red); if(closed) { order_count = 0; //break; } if(!closed) Alert("Trade not closed: ", pTicket); } else { Alert("Order not selected"); } }
-
OnTick is for EAs. OnStart is for scripts. You can't have both. Program Running - MQL4 programs - MQL4 Reference
-
Use the debugger or print out your variables, including _LastError and prices and find out why. Do you really expect us to debug your code for you?
Code debugging - Developing programs - MetaEditor Help
Error Handling and Logging in MQL5 - MQL5 Articles (2015)
Tracing, Debugging and Structural Analysis of Source Code - MQL5 Articles (2011)
Introduction to MQL5: How to write simple Expert Advisor and Custom Indicator - MQL5 Articles (2010) - Magic number only allows an EA to identify its trades from all others. Using OrdersTotal/OrdersHistoryTotal (MT4) or PositionsTotal (MT5), directly and/or no Magic number/symbol filtering on your OrderSelect / Position select loop means your code is incompatible with every EA (including itself on other charts and manual trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 programming forum (2013)
PositionClose is not working - MQL5 programming forum (2020)
MagicNumber: "Magic" Identifier of the Order - MQL4 Articles (2006)
Orders, Positions and Deals in MetaTrader 5 - MQL5 Articles (2011)
Limit one open buy/sell position at a time - General - MQL5 programming forum (2022)You need one Magic Number for each symbol/timeframe/strategy. Trade current timeframe, one strategy, and filter by symbol requires one MN.
Thank you for the response and sorry for expecting too much from this. I thot if somebody could help me it would be great. I am like a 2 year kid when it comes to coding. and i am trying to learn my way up.
thanks a lot for the help
Thank you for the response and sorry for expecting too much from this. I thot if somebody could help me it would be great. I am like a 2 year kid when it comes to coding. and i am trying to learn my way up.
thanks a lot for the help
Try this
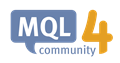
- docs.mql4.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi
I am a newbie into mql4 coding and i am trying do my first ever code for mt4. In my strategy I might have hedged positions, which I close all of them when it reaches break-even point. But unfortunately orderclose doesn't execute at all. In the backtesting orders are being opened but orders aren't closed. Can somebody help?
I am attaching the code here. Please advise.