Any questions from newcomers on MQL4 and MQL5, help and discussion on algorithms and codes - page 19

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
To correct this error, you need to understand your formula - what, why and how you are counting.
And only then you will be able to work out your algorithm and eliminate the error.
By "gut feeling" method and not understanding your formula - you will eliminate error, but you will make wrong calculation.
p.s. Explain in detail what you are calculating -- comment on your code in detail -- and then we will understand how you will fix the error.
We need an indicator which is based on Pearson's correlation coefficient values by close prices. We take an array of 24 bars (in my case it is variable n), starting from the first bar - for 2 currencies. Calculate the correlation for 24 bars - get value on the first bar. Then calculate the correlation for 24 bars, but starting from the second bar and so on.
1. I took a two-dimensional array of closing prices(i elements and p dimensions).
{
for(int p=0; p<m; p++)
{
Price_CloseX[i][p]=iClose(sym_x, PERIOD_H1, i+p);
Price_CloseY[i][p]=iClose(sym_y, PERIOD_H1, i+p);
}
}
{
for(int p=0; p<m; p++)
{
sum_x[i][p]=sum_x[i][p-1]+Price_CloseX[i][p];
sum_y[i][p]=sum_y[i][p-1]+Price_CloseY[i][p];
}
}
3. Average closing price over 24 bars(n-1) in each array
{
for(int p=0; p<m; p++)
{
Mx[i][p]=sum_x[p+1][m-1]/(n-1);
My[i][p]=sum_y[p+1][m-1]/(n-1);
}
}
4. the deviation from the average price in each array
{
for(int p=0; p<m; p++)
{
dx[i][p]=Price_CloseX[i][p]-Mx[i][p];
dy[i][p]=Price_CloseY[i][p]-My[i][p];
}
}
5. The square of the deviation from the average closing price
{
for(int p=0; p<m; p++)
{
dx2[i][p]=(dx[i][p]*dx[i][p]);
dy2[i][p]=(dy[i][p]*dy[i][p]);
}
}
6. The product of the deviations, i.e. each element in the currency 1 array is multiplied by the same element in currency 2,
e.g. the value of dx[1][0](currency 1) is multiplied by dy[1][0](currency 2); dx[3][4]*dy[3][4], etc.
{
for(int p=0; p<m; p++)
{
dxdy[i][p]=(dx[i][p]*dy[i][p]);
}
}
7. Calculate the sum of the squares of the deviations and the sum of the product of the deviations
{
for(int p=0; p<m; p++)
{
Edx2[i][p]=(Edx2[i-1][p]+dx2[i][p]);
Edy2[i][p]=(Edy2[i-1][p]+dy2[i][p]);
Edxdy[i][p]=(Edxdy[i-1][p]+dxdy[i][p]);
}
}
8. Well, actually the correlation coefficient and substitute it into the buffer
{
Koef[p]=Edxdy[n-1][p]/sqrt(Edx2[n-1][p]*Edy2[n-1][p]);
Buffer1[p]=Koef[p];
}
__________________________________________________________________________________________
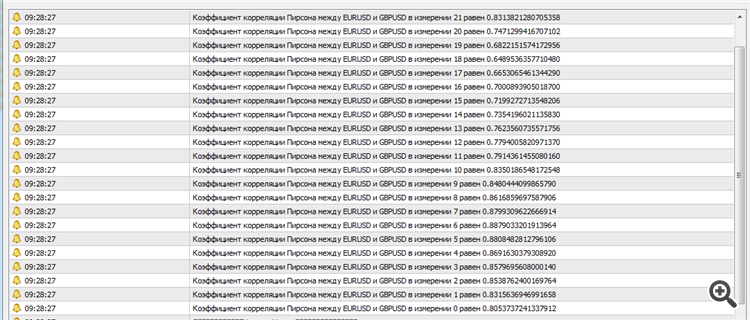
As written above, the error is an array overrun. The script with the same code for calculating the correlation coefficient considers this coefficient
When #property strict was written, the compiler generated errors in each for() loop saying that variables must be of type, so I had to write int i and int p in each loop. After that the compiler didn't generate errors, but the line didn't build. When I removed #property strict, the compiler did not require to declare the type in each loop, and the line was built.
int i and int p need only be declared once - before init()
you can write such a line before double Buffer1[];
in the int code remove
#property strict must be returned
then run the compilation again
then check for errors
then, in case of array overrun, look in which line of code (the number is of interest and the line itself). Earlier it was line number 90 in your code.
The error means that if you have 24 values in the array, and you request the 24th index, then . it's an error. Try to figure it out. The index numbering starts at zero, i.e. in your case 0,1,...23.
If we can not understand - throw this line here, we'll think
int i and int p need only be declared once - before init()
"The road to programming hell is paved with global variables". S. McConnell.
Sometimes it is more difficult without them, but not in the case of the indicator in question.
I agree, sometimes you can't do without them at all. But not in this case.
In this case, more code is written than is necessary and sufficient.
By the way, you can also write type before each variable even if the variable name is the same...., but it is not the right code construction.
In this case, more code is written than needed.
By the way, you can also write type before each variable, even if the variable name is the same...., but this is not the right code construction.
Actually I do, you probably shouldn't be advised to do so, but perhaps you will reconsider your decision when you encounter some problems.
...
In this case you have written more code than necessary and enough.
By the way, you can also write type before each variable, even if the variable name is the same...., but this is not the right code construction.