Hi I've encountered error code 1 when I'm trying to modify an order. I've looked into the web and the error occurred because the value is the same with the previous order modify. So I set a condition if there's no change of value in stoploss than return.
even after set in that condition the error code 1 still occurred. Is there's a way to fix the error code?
you are comparing two double values based on prices, it is unlikely that they will ever be an exact match, therefore your return statement is unlikely to ever get activated.
Use <= or >= instead of ==
correct your comparison so that it is not ==
make sure that you Normalize any calculated prices you send to the broker - https://www.mql5.com/en/docs/convert/normalizedouble
use the debugger or a print statement to look at what you are actually comparing and sending
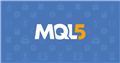
- www.mql5.com
Doubles are rarely equal. Understand the links in:
The == operand. - MQL4 programming forum #2 (2013)
If you are not moving the SL by at least a tick size, don't try.
Hi I've encountered error code 1 when I'm trying to modify an order. I've looked into the web and the error occurred because the value is the same with the previous order modify. So I set a condition if there's no change of value in stoploss than return.
even after set in that condition the error code 1 still occurred. Is there's a way to fix the error code?
You really should normalize the SL to tick size, but apart from that do you really want to modify your stop every point?
Some brokers really don't like it if your EA sends too many OrderModify().
I think that it is a good idea to use a step to prevent constant modifications.
Create a variable called step, maybe equal to 1 pip and then
(for a buy)
if(trailingStop-OrderStopLoss()>=step)
{
}
(for a sell)
if(OrderStopLoss()-trailingStop>=step || OrderStopLoss()=0) { }
Then you will have no problem with trying to modify the SL with the same value.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi I've encountered error code 1 when I'm trying to modify an order. I've looked into the web and the error occurred because the value is the same with the previous order modify. So I set a condition if there's no change of value in stoploss than return.
even after set in that condition the error code 1 still occurred. Is there's a way to fix the error code?