I want to declare these to variables in an EA:
int ema_lookback = 10;
double ema_wgt = (2 / (ema_lookback + 1));
When I declared both globally then ema_wgt was initialized as 0, and I found that a global variable can be initialized only by a constant. So then I declared ema_wgt globally and initialized locally but ema_wgt was still initialized as 0. So then I declared and initialized ema_wgt locally but ema_wgt was still initialized as 0. What do I need to do so that ema_wgt initializes properly based on ema_lookback?
provide full code if you need help.
or else https://www.mql5.com/en/docs/basis/variables/variable_scope
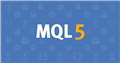
- www.mql5.com
provide full code if you need help.
or else https://www.mql5.com/en/docs/basis/variables/variable_scope
int ema_lookback = 10; double ema_wgt; void OnTick() { ema_wgt = (2 / (ema_lookback + 1)); Print("This is the ema_wgt: ", DoubleToString(ema_wgt,5)); }
Try
ema_wgt = (2.0 / (ema_lookback + 1));as you must have a double in the calculation or the result will be cast to an integer
rdone,
Please have a look at this article. In short don't use global variables unless you absolutely have to! When you do, by convention you should name them with the "g_" prefix so you don't mangle them in the namespace should you ever combine your source files into larger projects. For example:
g_global_variable; m_class_member_variable; s_static_variable; regular_variable;
In specific regard to your question, those should be local, with the possible exception of making the lookback const.
const int g_ema_lookback = 10; void OnTick() { double ema_wgt = (2.0 / (g_ema_lookback + 1)); Print("This is the ema_wgt: ", DoubleToString(ema_wgt,5)); }
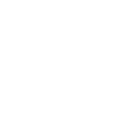
- www.learncpp.com
rdone,
Please have a look at this article. In short don't use global variables unless you absolutely have to! When you do, by convention you should name them with the "g_" prefix so you don't mangle them in the namespace should you ever combine your source files into larger projects. For example:
In specific regard to your question, those should be local, with the possible exception of making the lookback const.
Thanks Keith and Nocholi for lending a hand, much appreciated. With the decimal, ema_wgt will properly initialize globally or locally, and Nicholi's tip on naming conventions and declarations is useful coding hygiene.
Thanks Keith and Nocholi for lending a hand, much appreciated. With the decimal, ema_wgt will properly initialize globally or locally, and Nicholi's tip on naming conventions and declarations is useful coding hygiene.
The only time you should use a global variable in MQL is when you need a value to persists beyond the scope of the function, for example input constants, values set in OnInit and referred to later in other functions, etc. Other than that, don't do it!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I want to declare these to variables in an EA:
int ema_lookback = 10;
double ema_wgt = (2 / (ema_lookback + 1));
When I declared both globally then ema_wgt was initialized as 0, and I found that a global variable can be initialized only by a constant. So then I declared ema_wgt globally and initialized locally but ema_wgt was still initialized as 0. So then I declared and initialized ema_wgt locally but ema_wgt was still initialized as 0. What do I need to do so that ema_wgt initializes properly based on ema_lookback?