To answer my own question: I have a partial solution. This solution works in case the log file already exists. Everywhere in the code where I
currently use Alert() can I call this function.
What I don't know yet how to solve is:
(*) how to create a new log file if the log file doesn't exist yet?
(*) how to ensure that writing to file is only being done during paper/live trading, and not during back testing?
appendLogfile(string Line){ string Filename = Symbol()+”log.txt”; int handle = FileOpen(Filename, FILE_READ|FILE_WRITE|FILE_TXT);; if(handle > 0){ FileSeek(handle, 0, SEEK_END); FileWrite(handle, Line); FileClose(handle); } else{//file doesn’t exist, create a new one but how? } }
To answer my own question: I have a partial solution. This solution works in case the log file already exists. Everywhere in the code
where I currently use Alert() can I call this function.
What I don't know yet how to solve is:
(*) how to create a new log file if the log file doesn't exist yet?
(*) how to ensure that writing to file is only being done during paper/live trading, and not during back testing?
Typically you would just use Print and printf for that.
WindmillMQL:
(*) how to create a new log file if the log file doesn't exist yet?
(*) how to ensure that writing to file is only being done during paper/live trading, and not during back testing?
- The terminal's log file always exists and you use Print/Alert to add to it. If you mean your own file, it's not a log file (terminology matters; you confused nicholi with that.) Your posted code appends to the file or creates it if it doesn't yet exist. Perhaps you should read the manual.
- Test for it.
enum termnal_Mode{ MODE_DEBUG, MODE_LIVE, MODE_VISUAL, MODE_OPTIMIZER}; termnal_Mode get_modus_operandi(void){ if(IS_DEBUG_MODE) return MODE_DEBUG; // debugging. if(!MQLInfoInteger(MQL5_TESTING) ) return MODE_LIVE; // IsTesting() if( MQLInfoInteger(MQL5_VISUAL_MODE)() ) return MODE_VISUAL; // IsVisualMod return MODE_OPTIMIZER; }
- The terminal's log file always exists and you use Print/Alert to add to it. If you mean your own file, it's not a log file (terminology matters; you confused nicholi with that.) Your posted code appends to the file or creates it if it doesn't yet exist. Perhaps you should read the manual.
- Test for it.
1. Apparently I did cause confusion by using the word "log". That was unintentional. You are correct: I want to create my own file, not use the MT5 terminal's log file.
You mention that a file is automatically created if I try to open it with FileOpen() but it does not exist yet. Is this correct? I was under the
impression that if a file is non-existent FileOpen() will return INVALID_HANDLE. I did not see a statement that it will create a new file? If
Fileopen() returns an INVALID_HANDLE then I can not proceed to write text to that file. This reference document page did not clarify it for
me: https://www.mql5.com/en/docs/files/fileopen
2. Thank you for this code. I hadn't found those functions yet in the reference manual. I will read up about those in more detail.
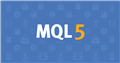
- www.mql5.com
Typically you would just use Print and printf for that.
I did not mean to append text to MT5 terminal's file. Instead I want to append text to my own text files. And want to create a file for each symbol which I use, so that the recorded text is separated for each symbol and not garbled together.
As I'm new in programming in MQL5 am I trying my way around in how to use files: create them, read them, write to them.
What part of "Your posted code appends to the file or creates it if it doesn't yet exist" was unclear? You didn't see it? You didn't drill down far
enough.
If FILE_READ is specified, an attempt is made to open an existing file. If a file does not exist, file opening fails, a new file is not created.FileOpen → Constants, Enumerations and Structures / Input/Output Constants / File Opening Flags - Reference on algorithmic/automated trading language for MetaTrader 5
FILE_READ|FILE_WRITE – a new file is created if the file with the specified name does not exist.
FILE_WRITE – the file is created again with a zero size.
What part of "Your posted code appends to the file or creates it if it doesn't yet exist" was unclear? You didn't see it? You didn't drill down
far enough.
Thank you for pointing that out to me. I had seen the page about File Opening Flags, which you link to, but had overlooked the sentence mentioning that if I specify "FILE_READ|FILE_WRITE" a new file will be created if it doesn't exist.
I will do some experiments to see how this works.- The terminal's log file always exists and you use Print/Alert to add to it. If you mean your own file, it's not a log file (terminology matters; you confused nicholi with that.) Your posted code appends to the file or creates it if it doesn't yet exist. Perhaps you should read the manual.
- Test for it.
I have a follow-up question on item #2. In the code you share you use the function IsTesting(). Is this a standard function? I could not find a reference to it in the MQL5 manual. If it is not a standard function, can you please share the code of this function with me?
Meanwhile have I been able to create new files, write text to it, have text overwritten and have text appended. I think that I have this portion of my
code now under control.
IsTesting - Checkup - MQL4 Reference
Migrating from MQL4 to MQL5 - MQL5 Articles № 6
It is in MT4. #3.2 adjusted for MT5 equivalents.
IsTesting - Checkup - MQL4 Reference
Migrating from MQL4 to MQL5 - MQL5 Articles № 6

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
So I have created my EA and am running it now as paper trading. In order to understand how it behaves does it contain various Alert() statements in the code. This helps me to understand when it detects certain signal changes, when it takes trades, print error messages (if any), how the account balance&equity values change over time, and so on. This works nicely but has one disadvantage: I run MT minimized on my computer but each time an Alert is placed the window pops up and interferes with the other work I'm doing. Each time I have to minimize the window and then it pops up again with the next message.
What I would like to do is to not use Alert() but to write these text messages to a text file, a log file. Then I can take a look at this log file at an appropriate time. Only for highly important messages would I then also use an Alert(). Each new message needs to be appended at the bottom of the file. So the oldest message is at the top and the latest message is at the end of the file.
Of course should this log file only be used during paper trading or live trading. It should not be used when in strategy testing (back testing) as this could result in enormously large text files.
My questions are: is it possible in MQL5 to write and append text to a text file? Where can I find examples of the basic setup of such a way of logging? And how can an EA identify whether it is being run in strategy testing, versus live/paper trading?