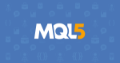
- www.mql5.com
- Invalid stops
- Invalid Stops in my EA Index
- MQL Storage Error on MT4
Well, we cannot say what is causing the error because we don't have the full code nor the execution conditions. Buy there're a few things I would like to point out:
1 - Multiplying the tp/sl by _Point is usually incorrect. The minimum price movement is a tick. So you probably want to call SymbolInfoDouble(_Symbol, SYMBOL_TRADE_TICK_SIZE).
2 - As it is on item 1, if _Point * value returns a value that is not a multiple of tick, you'll get an error (invalid price). That is because the entry price, tp and sl must be a multiple of tick. Considering this, moving averages usually are not normalized to tick. If you add MovingAverage + value, you need to normalize it so it is a multiple of tick. Doing it like this should be fine:
double Normalize(double value, double step) // step is the tick_size, value is the value to be normalized { return MathRound(value / step) * step; }
This should be done to the entry price, tp and sl. And maybe to the volume, if you do any math operation on it (step would be the volume step).
3 - It doesn't show in your code, but be sure the moving average is above the closing price when opening a sell position and below when opening a buy position. If not, invalid price may ocurr again, since the sl must be placed above the entry price when selling, and below it when buying.
So much is wrong here, so there are several things could be causing your issue.
I will try to touch on few main points that could cause you problems.
1)
Your entry price is just the last close price.
Since there's much of you code missing- who guarantee you that the last close price is the current market price? you don't show that you open a position on candle's start.
Also- Close price by default is either Ask or Bid (I currently can't remember which). But entry price for Buy and Sell is Ask and Bid respectively.
2)
This one is probably the one causing you the error message, but since it is also using the value of entry price (which I talked about above) I posted this second.
You are using the MA value plus some other variables to calculate the T/P and S/L.
What guarantees you that the T/P and S/L prices are actually above or below the current price?
For example the S/L calculation of MA - SLvsMA could still be higher than your open price (and the opposite for your T/P calculation).
I'm not an native English speaker, so I hope I've made my points clear enough.
Let me know if something isn't clear.
Cheers.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use