for(i=limit; i>0; i--)
Hello , please help me fix this..
i got an error while backtest ea for H1 timeframe but no problem for M1..
it say critical error in the EA, array out of range (1278,19)
when i compile there is no error sign..
i don't know how to fix this..
thank you
Array Out Of Range is a runtime (execution-time) error, it will never show up during compile time.
Basically your code structure is right - compile time, but it has a flaw in logic - rutime, as Marco already pointed.
You were trying to access an array element that does not exist. It is very common on languages using 0 (zero) as their first element on arrays...
hello, i try it but still error..
Array Out Of Range is a runtime (execution-time) error, it will never show up during compile time.
Basically your code structure is right - compile time, but it has a flaw in logic - rutime, as Marco already pointed.
You were trying to access an array element that does not exist. It is very common on languages using 0 (zero) as their first element on arrays...
limit=MathMin(limit,Bars-1-ExtDepth); for(i=limit; i>=0; i--)extremum=Low[iLowest(NULL,0,MODE_LOW,ExtDepth,i)]; ==> this is code number 1278
Try this, it may work for you.
Try this, it may work for you.
Then you need to show your code as we can only guess.
Then you need to show your code as we can only guess.
here it is,,
//----- double ZigZag(int l) { double ExtHighBuffer[]; double ExtLowBuffer[]; double ExtZigzagBuffer[]; //---- int NewSize=Zig_Zag_Back+1; //---- Set the direct indexing direction in the array ArraySetAsSeries(ExtZigzagBuffer,false); ArraySetAsSeries(ExtHighBuffer,false); ArraySetAsSeries(ExtLowBuffer,false); //---- Change the size of the emulated indicator buffers ArrayResize(ExtZigzagBuffer,NewSize); ArrayResize(ExtHighBuffer,NewSize); ArrayResize(ExtLowBuffer,NewSize); //---- Set the reverse indexing direction in the array ArraySetAsSeries(ExtZigzagBuffer,true); ArraySetAsSeries(ExtHighBuffer,true); ArraySetAsSeries(ExtLowBuffer,true); //--- globals int ExtLevel=3; // recounting's depth of extremums //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ int i=0,limit,counterZ,whatlookfor=0; int back,pos,lasthighpos=0,lastlowpos=0; double extremum; double curlow=0.0,curhigh=0.0,lasthigh=0.0,lastlow=0.0; //--- check for history and inputs if(Zig_Zag_Back<ExtDepth || ExtBackstep>=ExtDepth) return(0); //--- first calculations ArrayInitialize(ExtZigzagBuffer,0.0); ArrayInitialize(ExtHighBuffer,0.0); ArrayInitialize(ExtLowBuffer,0.0); //--- first calculations i=counterZ=0; //--- set start position to found extremum position limit=Zig_Zag_Back-ExtDepth; if(limit>Bars) limit=Bars-1; //--- main loop for(i=limit; i>0; i--) { //--- find lowest low in depth of bars extremum=Low[iLowest(NULL,0,MODE_LOW,ExtDepth,i)]; //--- this lowest has been found previously if(extremum==lastlow) extremum=0.0; else { //--- new last low lastlow=extremum; //--- discard extremum if current low is too high if(Low[i]-extremum>ExtDeviation*Point) extremum=0.0; else { //--- clear previous extremums in backstep bars for(back=1; back<=ExtBackstep; back++) { pos=i+back; if(ExtLowBuffer[pos]!=0 && ExtLowBuffer[pos]>extremum) ExtLowBuffer[pos]=0.0; } } } //--- found extremum is current low if(Low[i]==extremum) ExtLowBuffer[i]=extremum; else ExtLowBuffer[i]=0.0; //--- find highest high in depth of bars extremum=High[iHighest(NULL,0,MODE_HIGH,ExtDepth,i)]; //--- this highest has been found previously if(extremum==lasthigh) extremum=0.0; else { //--- new last high lasthigh=extremum; //--- discard extremum if current high is too low if(extremum-High[i]>ExtDeviation*Point) extremum=0.0; else { //--- clear previous extremums in backstep bars for(back=1; back<=ExtBackstep; back++) { pos=i+back; if(ExtHighBuffer[pos]!=0 && ExtHighBuffer[pos]<extremum) ExtHighBuffer[pos]=0.0; } } } //--- found extremum is current high if(High[i]==extremum) ExtHighBuffer[i]=extremum; else ExtHighBuffer[i]=0.0; } //--- final cutting if(whatlookfor==0) { lastlow=0.0; lasthigh=0.0; } else { lastlow=curlow; lasthigh=curhigh; } for(i=limit; i>=0; i--) { switch(whatlookfor) { case 0: // look for peak or lawn if(lastlow==0.0 && lasthigh==0.0) { if(ExtHighBuffer[i]!=0.0) { lasthigh=High[i]; lasthighpos=i; whatlookfor=-1; ExtZigzagBuffer[i]=lasthigh; } if(ExtLowBuffer[i]!=0.0) { lastlow=Low[i]; lastlowpos=i; whatlookfor=1; ExtZigzagBuffer[i]=lastlow; } } break; case 1: // look for peak if(ExtLowBuffer[i]!=0.0 && ExtLowBuffer[i]<lastlow && ExtHighBuffer[i]==0.0) { ExtZigzagBuffer[lastlowpos]=0.0; lastlowpos=i; lastlow=ExtLowBuffer[i]; ExtZigzagBuffer[i]=lastlow; } if(ExtHighBuffer[i]!=0.0 && ExtLowBuffer[i]==0.0) { lasthigh=ExtHighBuffer[i]; lasthighpos=i; ExtZigzagBuffer[i]=lasthigh; whatlookfor=-1; } break; case -1: // look for lawn if(ExtHighBuffer[i]!=0.0 && ExtHighBuffer[i]>lasthigh && ExtLowBuffer[i]==0.0) { ExtZigzagBuffer[lasthighpos]=0.0; lasthighpos=i; lasthigh=ExtHighBuffer[i]; ExtZigzagBuffer[i]=lasthigh; } if(ExtLowBuffer[i]!=0.0 && ExtHighBuffer[i]==0.0) { lastlow=ExtLowBuffer[i]; lastlowpos=i; ExtZigzagBuffer[i]=lastlow; whatlookfor=1; } break; } } //--- done
What about this ?
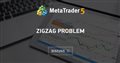
- 2014.11.09
- www.mql5.com
Why don't you just use buffers?
You are re-sizing the arrays to whatever and then trying to access the elements using i which is likely out of the array range.
I don't think that the error is where you showed earlier. I think that it is from
if(Low[i]==extremum) ExtLowBuffer[i]=extremum; else ExtLowBuffer[i]=0.0;
and similar.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello , please help me fix this..
i got an error while backtest ea for H1 timeframe but no problem for M1..
it say critical error in the EA, array out of range (1278,19)
when i compile there is no error sign..
i don't know how to fix this..
thank you