Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Fernando Carreiro #:
Your topic has been moved to the section: Technical Indicators
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Given that your rating is still too, you will not be able to embed images. You can however attach images as files using the "+ Attach" link just below the posting area.
Can you explain how you are colouring the candles?
Show a sample of the code that you are using for it. Are you using the "DRAW_COLOR_CANDLES" or something else?
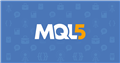
- www.mql5.com
Can you explain how you are colouring the candles?
Show a sample of the code that you are using for it. Are you using the "DRAW_COLOR_CANDLES" or something else?
Then, please note that the "DRAW_COLOR_CANDLES" (or the "DRAW_CANDLES") method does not have an option for "hollow" candles.
They are always "filled". That is to say that the border and fill are always the same colour, unlike the regular candles which can have separate colours.
Then, please note that the "DRAW_COLOR_CANDLES" (or the "DRAW_CANDLES") method does not have an option for "hollow" candles.
They are always "filled". That is to say that the border and fill are always the same, unlike the regular candles which can have separate colours.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have written an indicator that colors candles based on The Strat method. The indicator works as I intended coloring the 1 candles yellow and they 3 candles Magenta. Unfortunately this overrides the chart's default settings for Bull/Bear candles. I like for my Bull candles to be hollow and I am able to achieve this affect for candles not painted by the indicator by setting the Bull Candle color to the same color as my background in the chart properties, but after searching the documentation, I cannot find the right property to do this from within my code. The end result is pictured below here in as you can see, the green bull candles appear to be hollow as I want, but the candles which my inicator paints are solid regardless of direction. Both the yellow (1) and magenta (3) candles in the middle of this chart should be hollow. How can I achieve this?
EDIT#2: Chart image attached as advised.
EDIT#3: Adding code for reference.