Hi,
i try to understand the basic things in mql5
So at the moment i want to define a array, for that i do this
now i want to store and return some different kinds of datas
in the first element should a integer value
in the second a double value
At the moment all values returns "0.00" (= double).
I thought double arrays can store integer values too?
Have i do something more to get what i want?
Thanks to make it clear for me ;-)
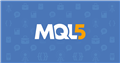
- www.mql5.com
Hello,
if I understand that correctly, is there no way to declare the memory space of the individual array elements as an "integer" type?
I need to specifically format the output of the array element instead? This would mean, however, that this formatting must take place always and everywhere, if the value is saved directly and correctly, this effort would not be necessary.
Isn't there such a possibility or did I misunderstand something? If in doubt, maybe someone can give me a short example of my code above, then it might explain it better? Thanks!
Hi,
i try to understand the basic things in mql5
So at the moment i want to define a array, for that i do this
now i want to store and return some different kinds of datas
in the first element should a integer value
in the second a double value
Use a struct array.
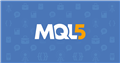
- www.mql5.com
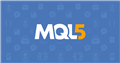
- www.mql5.com
You are a long time poster, but you still don't post code properly. Please do so with the "</>" icon or Alt-S.
You are a long time poster, but you still don't post code properly. Please do so with the "</>" icon or Alt-S.
You are right, it is better:
//+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- double array[] = {1.234, 45.67, 789.0}; for(int i = 0; i < ArraySize(array) && !IsStopped(); i++) Print((int)array[i]); //Results: //1 //45 //789 }(Fixed some errors btw)
i think this is my favorite way.
Is it possible to work with this in/for a function?
Can i give this array to a function, if is there a special way or only like above?
void my_function(my_struct_array)
{
//do i haveto return it or can I access of the proceesed values outside from the function to?
}
i think this is my favorite way.
Is it possible to work with this in/for a function?
Can i give this array to a function, if is there a special way or only like above?
void my_function(my_struct_array)
{
//do i haveto return it or can I access of the proceesed values outside from the function to?
}
You can pass it by reference.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
i try to understand the basic things in mql5
So at the moment i want to define a array, for that i do this
now i want to store and return some different kinds of datas
in the first element should a integer value
in the second a double value
At the moment all values returns "0.00" (= double).
I thought double arrays can store integer values too?
Have i do something more to get what i want?
Thanks to make it clear for me ;-)