#include <Arrays/ArrayDouble.mqh> CHashMap<int, CArrayDouble *> Map;I don't think it works with native arrays. You could try this.
CArrayDouble *
I want to declare a function that uses this hashmap as its parameter/arguments.
My function declaration is like...below: (I am not sure if its the correct way to declare, please correct me if i am wrong)
bool doSomething(CHashMap<int, CArrayDouble *> &infoMap){ ... }
In above case i got error: Expression are not allowed in global scope.
I also tried declaring functin like this below and got error: CHAshMap: Objects are passed by reference only
bool doSomething(CHashMap<int, CArrayDouble *> infoMap){ ... }
I Am not sure how to use CArrayDouble in a hashmap as part of functino declaration, as well as function calling to extract the values from the CArrayDouble while iterating through the Hashmap... . When I was extracting values from Hashmap without CArrayDouble I used the below code:
map.CopyTo(x, y); a = y;
Also When i tried to declare in global scope:
CHashMap<int, CArrayDouble *> Map;
I get error Expression are not allowed in global scope.
Can you please guide with the usage in above cases? Thank you
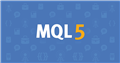
- www.mql5.com
It's your task to get informed about the usage of CHashMap/CArrayDouble. Please consult the manual and study the .mqh files.
#include <Generic/HashMap.mqh> #include <Arrays/ArrayDouble.mqh> CHashMap<int,CArrayDouble *>Map; // bool doSomething(CHashMap<int,CArrayDouble *>&infoMap) { infoMap.Clear(); CArrayDouble *values=new CArrayDouble; values.Add(0.1); values.Add(0.2); int key=4711; infoMap.Add(key,values); return true; } // int OnInit() { doSomething(Map); CArrayDouble *values; if(Map.TryGetValue(4711,values)) { Print("got ",values.Total()," values"); } ...
Thank you! Those errors are gone. I will do more reading to understand these classes as well as pointers. I was going through this link to understand pointers: https://www.mql5.com/en/docs/basis/types/object_pointers
1. However I am a bit confused about usage of pointers inside the CHashMap. Should i delete it CArrayDouble somehow and somewhere as it is a pointer?
Or is it not needed? The website page said we should delete them in the end.
2. Are there any advantages using the pointer and non pointer declaration? In which case is it required to declare using pointer decleration? (with *)
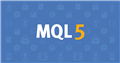
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi,
I want to create a HashMap which has following declaration but it gives error. "
CHashMap<int, float[]> x;
Error :
'[' - comma expected "
Is there a way to have hashmap with key as int and value as an array? So that I can store multiple values for a single key.