This indicator requires some values of ADX on the setting panel, but it is actually calculating CCI. I have fixed some parts and added alerts.
This indicator requires some values of ADX on the setting panel, but it is actually calculating CCI. I have fixed some parts and added alerts.
thank you . and alert comes with symbol of chart i use ,not symbol that actually generated alert
i changed SYMBOL()to symbol name but no difference see THE IMAGES
Line 232
DrawLabel("cci_" + symbolName, x, y, GetAdxStr(CCI, CCIY, symbolName), fontSize, fontName, GetAdxColor(CCI, CCIY), tooltip);
Line 237
string GetAdxStr(double CCI, double CCIY, string symbolName)
Line 241, 247
Do_Alert(" Buy Signal", symbolName); Do_Alert(" Sell Signal", symbolName);
Line 442
void Do_Alert(string doWhat, string symbolName)
thanks . but it remains the same i can adjust with that.
now if buy signal from one and sell signal from another happens at same time .the alert is given continuously every second .can u solve this please
image attached
To do that, you have to set up and manage an index for each currency pair.
But it's impossible because the original program does not have it.
If a timer is used, an approximate method can be used.
Line 15
input int TimerInterval = 5; //Update interval (minutes)
Line 70
EventSetTimer(TimerInterval * 60);
thanks . but it remains the same i can adjust with that.
now if buy signal from one and sell signal from another happens at same time .the alert is given continuously every second .can u solve this please
image attached
you only need to open a job in the job section
then your problem will soon be resolved
:)
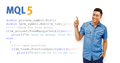
- www.mql5.com
it can be fixed
you only need to open a job in the job section
then your problem will soon be resolved
:)
thanks man .before this i didn't know it even existed

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use