-
Please edit your (original) post and use the CODE button (Alt-S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum (2019)
Messages Editor - Chris Pinter: from 1.05311 to 1.05310 (error 10016) . The last two lines show the stoploss was able to be modified to 1.05309.
if(anew_sl > aold_sl) // if the new sl is greater then we can change it
Your posted code would not even try to modify your sell position.
-
Please edit your (original) post and use the CODE button (Alt-S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum (2019)
Messages Editor -
Your posted code would not even try to modify your sell position.
1. I did use the code button to post code. Thanks
2. The code you pointed to does this special thing which is ... "if the new stoploss is greater than the old stoploss. " if you look to the right of the code you can see even more information
if(anew_sl > aold_sl) // if the new sl is greater then we can change it
It also means... if the new stoploss is less than the old stoploss dont do the following code. Can you see this?
I guess I could add an else statement that does nothing ( or return)...but that would be redundant.
I am getting a weird issue with modifying a position stoploss. Sometimes the stoploss cannot be changed and sometimes it does not. I am running this code on the Strategy Tester....but it also happens in real trading.
Here is the error message I generate. Most of the lines in this message log is created by the function below. However, The second line and the very last line are generated by the strategy tester
This code has two modify commands listed . The first three lines show that the operation failed to change the stoploss from 1.05311 to 1.05310 (error 10016) . The last two lines show the stoploss was able to be modified to 1.05309.
This is the code I am using the change the Stoploss. At one point during troubleshooting, I thought it might be a latency issue between my computer and the server computer. However, I am using the Strategy tester so it cannot be latency between two machines.
Any ideas why this is giving me grief?
Is the stop level limit of the brokerage checked ?
In the code below , its a quick copy paste of a check and enforcement of a limit for the opening of a new buy order with the take profit stop .
If i recall correctly the limitation has to do with distance from the live price
MqlTick tick; bool GetTick=SymbolInfoTick(symbol,tick); //preparation point=SymbolInfoDouble(symbol,SYMBOL_POINT); digits=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //get the limit in points stoplimit=(int)SymbolInfoInteger(symbol,SYMBOL_TRADE_STOPS_LEVEL); //turn to price limit_price=NormalizeDouble(((double)stoplimit*point),digits); //if there is no limit it will be 0.0 anyway , if there is we will get one and a half times as the applied stop size in case we //breach it additive_price=NormalizeDouble((limit_price*1.5),digits); //enforcement double dist=(take_profit_as_price-tick.ask); if(dist<=limit_price) take_profit_as_price=tick.ask+additive_price;
I am getting a weird issue with modifying a position stoploss. Sometimes the stoploss cannot be changed and sometimes it does not. I am running this code on the Strategy Tester....but it also happens in real trading.
Here is the error message I generate. Most of the lines in this message log is created by the function below. However, The second line and the very last line are generated by the strategy tester
This code has two modify commands listed . The first three lines show that the operation failed to change the stoploss from 1.05311 to 1.05310 (error 10016) . The last two lines show the stoploss was able to be modified to 1.05309.
This is the code I am using the change the Stoploss. At one point during troubleshooting, I thought it might be a latency issue between my computer and the server computer. However, I am using the Strategy tester so it cannot be latency between two machines.
Any ideas why this is giving me grief?
Read the Documentation How to edit SL and TP.
Your code is not complete, but I tried it and I got erros when getting/selecting Position Ticket and if the new stoploss was not properly set such as above open price of a buy position or below open price of a sell position.
I wonder you set the new SL properly and its digits or getting ea magic or getting the old SL since your code was not complete
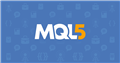
- www.mql5.com
In the first picture you attached you received the invalid stop loss error when you tried to modify your stop loss to the initial value. The second time new value was different and it succeeded. My guess: you should check the old and new values and only try to modify stop loss if they are not the same.
Read the Documentation How to edit SL and TP.
Your code is not complete, but I tried it and I got erros when getting/selecting Position Ticket and if the new stoploss was not properly set such as above open price of a buy position or below open price of a sell position.
I wonder you set the new SL properly and its digits or getting ea magic or getting the old SL since your code was not complete
Read the Documentation How to edit SL and TP.
Your code is not complete, but I tried it and I got erros when getting/selecting Position Ticket and if the new stoploss was not properly set such as above open price of a buy position or below open price of a sell position.
I wonder you set the new SL properly and its digits or getting ea magic or getting the old SL since your code was not complete
I have set the SL to a specific set of digits using NormalizeDouble()...see line 3 and 4 of the function.
How is the code not complete? Please be specific so I can learn.
limit_price=NormalizeDouble(((double)stoplimit*point),digits);
-
A number times point is not a price. 10 * 0.00001 = 0.00010. SL/TP are prices; not a change in price, a distance.
-
You used NormalizeDouble, It's use is usually wrong, as it is in your case.
-
Floating point has a infinite number of decimals, it's your not understanding floating point and that some numbers can't be represented exactly. (like 1/10.)
Double-precision floating-point format - Wikipedia, the free encyclopediaSee also The == operand. - MQL4 programming forum (2013)
-
Print out your values to the precision you want with DoubleToString - Conversion Functions - MQL4 Reference.
-
SL/TP (stops) need to be normalized to tick size (not Point) — code fails on non-currencies.
On 5Digit Broker Stops are only allowed to be placed on full pip values. How to find out in mql? - MQL4 programming forum (2011)And abide by the limits Requirements and Limitations in Making Trades - Appendixes - MQL4 Tutorial and that requires understanding floating point equality Can price != price ? - MQL4 programming forum (2012)
-
Open price for pending orders need to be adjusted. On Currencies, Point == TickSize, so you will get the same answer, but it won't work on non-currencies. So do it right.
Trailing Bar Entry EA - MQL4 programming forum (2013)
Bid/Ask: (No Need) to use NormalizeDouble in OrderSend - MQL4 programming forum (2012) -
Lot size must also be adjusted to a multiple of LotStep and check against min and max. If that is not a power of 1/10 then NormalizeDouble is wrong. Do it right.
(MT4 2013)) (MT5 2022)) -
MathRound() and NormalizeDouble() are rounding in a different way. Make it explicit.
MT4:NormalizeDouble - MQL5 programming forum (2017)
How to Normalize - Expert Advisors and Automated Trading - MQL5 programming forum (2017) -
Prices you get from the terminal are already correct (normalized).
-
PIP, Point, or Tick are all different in general.
What is a TICK? - MQL4 programming forum (2014)
-

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am getting a weird issue with modifying a position stoploss. Sometimes the stoploss cannot be changed and sometimes it does not. I am running this code on the Strategy Tester....but it also happens in real trading.
Here is the error message I generate. Most of the lines in this message log is created by the function below. However, The second line and the very last line are generated by the strategy tester
This code has two modify commands listed . The first three lines show that the operation failed to change the stoploss from 1.05311 to 1.05310 (error 10016) . The last two lines show the stoploss was able to be modified to 1.05309.
This is the code I am using the change the Stoploss. At one point during troubleshooting, I thought it might be a latency issue between my computer and the server computer. However, I am using the Strategy tester so it cannot be latency between two machines.
Any ideas why this is giving me grief?
void modify_eurusd_buy_position(double xanew_sl, double xaold_sl, long a_ticket, double c_price, double jstLoss) { double aold_sl; double anew_sl; aold_sl = NormalizeDouble(xaold_sl,5); anew_sl = NormalizeDouble(xanew_sl,5); if ((anew_sl == aold_sl) || (anew_sl < aold_sl)) { return; } if(anew_sl > aold_sl) // if the new sl is greater then we can change it { if (anew_sl != aold_sl) { s_current_price = DoubleToString(c_price, 5); s_breakeven = DoubleToString(TSL_one_eurusd, 5); string sa_itp_eurusd = DoubleToString(tp_eurusd,5); string s_old_sl = DoubleToString(aold_sl, 5); string s_new_sl = DoubleToString(anew_sl, 5); string s_jstLoss = DoubleToString(jstLoss, 1); if((anew_sl + aold_sl) == 0){return;} // mql5 is sending multiple statements PositionSelectByTicket(a_ticket); // get the right ticket, PositionGetInteger, PositionGetDouble and PositionGetString can now be used. MqlTradeRequest arequest; MqlTradeResult aresult; ZeroMemory(arequest); ZeroMemory(aresult); arequest.action =TRADE_ACTION_SLTP; // type of trade operation arequest.type_filling = ORDER_FILLING_IOC; // Order execution type arequest.position= a_ticket; // ticket of the position arequest.sl = anew_sl; arequest.tp = 0.0000; arequest.magic = EA_Magic; //--- send the request if(!OrderSend(arequest,aresult)) { if(aresult.retcode!=10009) { if(aresult.retcode!=10025) { Print(""); PrintFormat(" -- Position modify error | ticket : %i | error: %d , retcode=%u ", a_ticket, GetLastError(), aresult.retcode); // if unable to send the request, output the error code } } else { PrintFormat ("Ticket: %i | (%s) Modify SL: %s --> %s | Trail SL: %s | TP: %s | Current Price: %s ", a_ticket, s_jstLoss, s_old_sl, s_new_sl, s_breakeven, sa_itp_eurusd, s_current_price); } } } return; } }