Discussing the article: "Indicator of historical positions on the chart as their profit/loss diagram"
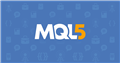
- www.mql5.com
It is unfortunate that MQ did not add the HistoryPositionGet-function to the list of History -functions. It would have been much easier with it.
That's why we have to do it ourselves
Hello,
how can I make the indicator show not every profit/loss, but an accumulative total ?
and if possible - with a line ?!!
Hello,
how can I make the indicator show not every profit/loss, but the cumulative total ?
and if possible - with a line ?!!
On a hunch and without checking, you just need to add the profit to the already received one, and not to record it every time the one taken from the positions.
That'show it is:
//+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- Устанавливаем индексацию массивов close и time как в таймсерии ArraySetAsSeries(close,true); ArraySetAsSeries(time,true); //--- Флаг успешного создания списка позиций static bool done=false; //--- Если объект данных позиций создан if(history!=NULL) { //--- Если ещё не создавался список позиций if(!done) { //--- Если список позиций по текущему инструменту успешно создан, if(history.CreatePositionList(Symbol())) { //--- распечатываем в журнале позиции и устанавливаем флаг успешного создания списка позиций history.Print(); done=true; } } } //--- Количество баров, необходимое для расчёта индикатора int limit=rates_total-prev_calculated; //--- Если limit больше 1 - значит это первый запуск или изменение в исторических данных if(limit>1) { //--- Устанавливаем количество баров для расчёта, равное всей доступной истории и инициализируем буферы "пустыми" значениями limit=rates_total-1; ArrayInitialize(BufferFilling1,EMPTY_VALUE); ArrayInitialize(BufferFilling2,EMPTY_VALUE); } //--- В цикле по барам истории символа static double profit=0; for(int i=limit;i>=0;i--) { //--- получаем профит позиций, присутствующих на баре с индексом цикла i и записываем в первый буфер полученное значение profit+= Profit(close[i],time[i]); BufferFilling1[i]=profit; //--- Во второй буфер всегда записываем ноль. В зависимости от того, больше или меньше нуля значение в первом буфере, //--- будет меняться цвет рисуемой заливки между массивами 1 и 2 буфера индикатора BufferFilling2[i]=0; } //--- return value of prev_calculated for next call return(rates_total); }
Well, and with the buffer as a line - it's themselves. There you need to remove one extra buffer, because the fill always uses two buffers. And for a line you need one.
I think it's working.
I made your changes and just specified the type - line graphics.
I didn't remove the buffers.
https://www.mql5.com/ru/charts/18738352/nzdcad-d1-roboforex-ltd
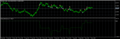
- www.mql5.com
Everything seems to be fine on currencies, but on silver it is constantly redrawing for some reason.
It is necessary to look at what data the indicator receives and why it is not calculated. The redrawing can be caused by the limit value greater than 1. This value is calculated as the difference between rates_total and prev_calculated. You need to look at these values to see what they contain on each tick

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Check out the new article: Indicator of historical positions on the chart as their profit/loss diagram.
In this article, I will consider the option of obtaining information about closed positions based on their trading history. Besides, I will create a simple indicator that displays the approximate profit/loss of positions on each bar as a diagram.
The function gets the price (bar's Close), relative to which the number of profit points of the position should be obtained, as well as the time, at which the existence of the position is checked (bar's Time open). Next, the profit of all positions received from each object of historical positions is summed up and returned.
After compilation, we can run the indicator on the chart of a symbol where there were many open positions, and it will draw a profit chart of all historical positions:
Author: Artyom Trishkin