Which MT5 Build do you have ?
https://www.mql5.com/en/forum/445154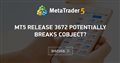
- 2023.04.08
- www.mql5.com
Hi Alain,
I have MT5 build 3674. I believe the latest version. So if it's the build, is there a way to role back maybe. What would you suggest please?
Thanks for the quick response Alain.
Kind regards,
Shep
Hi Alain,
Quick update. I rolled back the installation to an earlier build and my code compiles now. At least now I understand the reason for the compilation failures and now need to develop a plan to deal with these potential errors caused by updates - maybe consider stopping automatic updates.
Thanks for help.
Shep
I have the suspicion that MQ-demo accounts are automatically updated (or annoying by request window) no matter whether you have defined to use only release versions - but not demo and real accounts of real brokers.
Forum on trading, automated trading systems and testing trading strategies
General rules and best practices of the Forum.
Alain Verleyen, 2023.04.09 13:27
Official releases always have a public announcement.
It's the broker who decided which release to push to their clients.
The betas are entirely Metaquotes responsibility (brokers can't push them). The main server for betas is MetaQuotes-demo. This server is used by Metaquotes when they approach the release dates to conduct larger beta tests, as they know a lot of active developers use it for their tests.
If you don't want to be a beta-tester, never use MetaQuotes-demo server.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi all,
A few moments ago I restarted the MT5 platform on request for an update and upon starting Metaeditor5, all my classes with template<typename Object> have stopped working I get
"'Object' - unexpected token, probably type is missing? Objects.mqh 171 1'*' - semicolon expected Objects.mqh".
I have not done anything at all except restart the platform. I wrote the container below years ago, and now it has suddenly started bugging out. This is rather disconcerting. I would really appreciate any advice. Has anyone faced this problem before, and how can I resolve it?
Thank you all in advance,
Shep