- The formula to calculate the Exponential moving average = [Close - previous EMA] * (2 / n+1) + previous EMABe careful with precedence. The formula is c=p+(v-p) * 2/(n+1). You wrote p+(v-p) * (1 + 2/n) but correctly calculated 2/(n+1). Never used the equation 'c=(1-a)p + (a)v', as it amplifies round off errors.
-
double arr[3] = {1.3051,1.3041,1.3047};//last three bars' closing prices array double ema1 = iMAOnArray2(arr,2,MODE_EMA,0);// result is 1.30476667
Be careful with ordering. Since you didn't set the direction (SetAsSeries) of your array, OnArray is assuming that 1.3051 (index zero) is the latest value. [(1.3051 - 1.3041) * (2 / 2+1)] + 1.3041 = [0.001 * (2/3)] + 1.3050 = 1.30476666667
You are calculating as if 1.3041 was the previous EMA value. It is not.- In the exponential moving average (EMA,) the initial value is some what arbitrary, even a constant of zero works given enough future bars. To get within 0.1 percent of true value requires 3.45 * (Length+1) bars. To get an accurate average sooner requires a better initial value. Metaquotes uses SMA(L). Still, don't expect a valid EMA(2) until you have at least 10 bars.
1.3050 shift=0 1.3051 shift=1 (I am using this shift) 1.3041 shift=2 1.3047 shift=3
Your calculation should be- EMA4=(1.3047+1.3041)/2=1.3044
- EMA3=1.3044+2/3(1.3047-1.3044)=1.3046
- EMA2=1.3046+2/3(1.3041-1.3046)=1.304266666666667
- EMA1=1.304266666666667+2/3(1.3051-1.30426666666666771=1.304822222222222
"Be careful with ordering. Since you didn't set the direction (SetAsSeries) of your array, OnArray is assuming that 1.3051 (index zero) is the latest value."
"Metaquotes uses SMA(L)"
You can not check averages like EMA using just 3 values in an array *the iMAOnArray() call) and compare it to EMA that had (nnn) previous values already calculated (the iMA() call). It is not like SMA
EMA needs to "build up" and you have to have a sample larger than that to get "stable" EMA values. Check here about the EMA calculation : Moving Average (scroll down will you find the "Approximating the EMA with a limited number of terms" part)
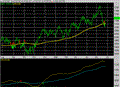
- en.wikipedia.org
Not always. You missed the point.
- In MT4, buffers and the predefined arrays are all ordered AsSeries. All others can be either direction. There is a difference between the arrays passed to OnCalculate (e.g. low[]) and the MT4 predefined variables (e.g. Low[].) The The passed arrays have no default direction.
- In MT5, you must set the direction.
To define the indexing direction in the time[], open[], high[], low[], close[], tick_volume[], volume[] and spread[] arrays, call the ArrayGetAsSeries() function. In order not to depend on defaults, call the ArraySetAsSeries() function for the arrays to work with.
Event Handling / OnCalculate - Reference on algorithmic/automated trading language for MetaTrader 5

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Returns a result of "1.30487345". Now my function returns "1.30476667" and I can't figure out why the result is different if both functions are correct.
Here is my function:
I decided to manually copy the chart's data set the iMA function is using and I observed the following closing prices: