- No need for the if.
openedtrades=0; for (int i=OrdersTotal() - 1; i>=0; --i) {
- Get in the habit of always counting down.In the presence of multiple orders (one EA multiple charts, multiple EAs, manual trading,) while you are waiting for the current operation (closing, deleting, modifying) to complete, any number of other operations on other orders could have concurrently happened and changed the position indexing:
-
For non-FIFO (US brokers,) (or the EA only opens one order per symbol,) you
can simply count down in a position loop, and you won't miss
orders. Get in the habit of always counting down.
Loops and Closing or Deleting Orders - MQL4 and MetaTrader 4 - MQL4 programming forum
For FIFO (US brokers,) and you (potentially) process multiple orders per symbol, you must count up and on a successful operation, reprocess all positions (set index to -1 before continuing.) -
and check OrderSelect in case earlier positions were deleted.
What are Function return values ? How do I use them ? - MQL4 and MetaTrader 4 - MQL4 programming forum
Common Errors in MQL4 Programs and How to Avoid Them - MQL4 Articles - and if you (potentially) process multiple orders, must call RefreshRates() after server calls if you want to use the Predefined Variables (Bid/Ask) or OrderClosePrice() instead, on the next order/server call.
-
For non-FIFO (US brokers,) (or the EA only opens one order per symbol,) you
can simply count down in a position loop, and you won't miss
orders. Get in the habit of always counting down.
- Make Total_Average_Price a local variable. It's an intermediary, it's neither an average nor a price. Average price is your break even.
- No Magic number filtering on your
OrderSelect loop means your code is incompatible with every EA (including itself on other charts and manual
trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 and MetaTrader 4 - MQL4 programming forum - Your problem is elsewhere.
void counttrades() { //---- openedtrades=0; lastticket = 0; for (int i=0; i<OrdersTotal(); i++) { if (!OrderSelect(i,SELECT_BY_POS)) continue; if (OrderSymbol()!=Symbol()) continue; if( OrderType()!=ORDER_TYPE_BUY && OrderType()!=ORDER_TYPE_SELL ) continue; openedtrades++; total_average_price+=(OrderOpenPrice()*OrderLots()); total_lots+=OrderLots(); breakeven_price=(total_average_price/total_lots); if(lastticket > OrderTicket()) continue; lastticket=OrderTicket(); lastopenlots=OrderLots(); lastopentype=OrderType(); lastopenprice=OrderOpenPrice(); } }
Or
void counttrades() { //---- openedtrades=0; datetime timeOrderOpen = 0; for (int i=0; i<OrdersTotal(); i++) { if (!OrderSelect(i,SELECT_BY_POS)) continue; if (OrderSymbol()!=Symbol()) continue; if( OrderType()!=ORDER_TYPE_BUY && OrderType()!=ORDER_TYPE_SELL ) continue; openedtrades++; total_average_price+=(OrderOpenPrice()*OrderLots()); total_lots+=OrderLots(); breakeven_price=(total_average_price/total_lots); if(timeOrderOpen > OrderOpenTime()) continue; timeOrderOpen = OrderOpenTime(); lastticket=OrderTicket(); lastopenlots=OrderLots(); lastopentype=OrderType(); lastopenprice=OrderOpenPrice(); } }
Or
Thank you very much, brother! appreciate for your suggestion. will certainly try them. Thanks once again.
- No need for the if.
- Get in the habit of always counting down.
- Make Total_Average_Price a local variable. It's an intermediary, it's neither an average nor a price. Average price is your break even.
- No Magic number filtering on your
OrderSelect loop means your code is incompatible with every EA (including itself on other charts and manual
trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 and MetaTrader 4 - MQL4 programming forum - Your problem is elsewhere.
Appreciate your sharing. Thank you for teaching, and suggestion.
I am actually searching and trying to write an EA to help me to calculate
1. Breakeven Point + few pips profit.
2. Modify my trades to the price above.
These trades are manually entered, that's why when at the loop, there is no filter for Magicnumber.
After few tries, is not success yet, and will try again and again. Thanks guys.
if you ever come across anything similar, hope you don't mind sharing the link or articles where i can study deeper.
I am actually searching and trying to write an EA to help me to calculate
1. Breakeven Point + few pips profit.
2. Modify my trades to the price above.
These trades are manually entered, that's why when at the loop, there is no filter for Magicnumber.
After few tries, is not success yet, and will try again and again. Thanks guys.
if you ever come across anything similar, hope you don't mind sharing the link or articles where i can study deeper.
Here is some ideas.
Just loop the OrdersTotal() and get OpenPrice & OrderLot of each order, plus Sum of OrderLot.
- a) if total order = 1, breakeven price = open price.
b) if total order > 1, breakeven price = ((orderlot1*openPrice1+orderlot2*openPrice2+....)*pipvalue) / totalOrderLot. - Modify order, based on order direction. (buy/sell), above / below.
good luck.
ps: @Konstantin Nikitin did provide the code for breakeven_price, perhaps breakeven_price=((total_average_price*pipsvalue)/total_lots)
Here is some ideas.
Just loop the OrdersTotal() and get OpenPrice & OrderLot of each order, plus Sum of OrderLot.
- a) if total order = 1, breakeven price = open price.
b) if total order > 1, breakeven price = ((orderlot1*openPrice1+orderlot2*openPrice2+....)*pipvalue) / totalOrderLot. - Modify order, based on order direction. (buy/sell), above / below.
good luck.
ps: @Konstantin Nikitin did provide the code for breakeven_price, perhaps breakeven_price=((total_average_price*pipsvalue)/total_lots)
Dear Mohamad,
I want to thank you for pointing out a direction where i can follow. Hope you don't mind asking you a question.
I understand most of your given advice accept this "pipvalue". Do you have little more info about pipvalue i can refer to?
Dear Mohamad,
I want to thank you for pointing out a direction where i can follow. Hope you don't mind asking you a question.
I understand most of your given advice accept this "pipvalue". Do you have little more info about pipvalue i can refer to?
if your deposit currency is in USD, and you trade USD based pair, example EURUSD, GBPUSD... pipsvalue = 1.
else if you trade other pair with different USD based currency, you need to accurately calculate every pip( point) movement. example, EURJPY, USDJPY etc...
double pipsvalue = SymbolInfoDouble(_Symbol,SYMBOL_TRADE_TICK_VALUE);
https://docs.mql4.com/constants/environment_state/marketinfoconstants
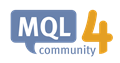
- docs.mql4.com
- Pip:Using Point means code breaks on 4 digit brokers, exotics (e.g. USDZAR where spread is over 500 points,) and metals. Compute what a PIP is and use it, not points.
How to manage JPY pairs with parameters? - MQL4 and MetaTrader 4 - MQL4 programming forum
Slippage defined in index points - Currency Pairs - Expert Advisors and Automated Trading - MQL5 programming forum - Delta: Value per price change per lot:Risk depends on your initial stop loss, lot size, and the value of the pair.
- You place the stop where it needs to be - where the reason for the trade is no longer valid. E.g. trading a support bounce the stop goes below the support.
- Account Balance * percent/100 = RISK = OrderLots * (|OrderOpenPrice - OrderStopLoss| * DeltaPerLot + CommissionPerLot) (Note OOP-OSL includes the SPREAD, and DeltaPerLot is usually around $10/pip but it takes account of the exchange rates of the pair vs. your account currency.)
-
Do NOT use TickValue by itself - DeltaPerLot
and verify that MODE_TICKVALUE is returning a value in your deposit
currency, as promised by the documentation, or whether it is returning a value
in the instrument's base currency.
MODE_TICKVALUE is not reliable on non-fx instruments with many brokers. - You must normalize lots properly and check against min and max.
- You must also check FreeMargin to avoid stop out

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am trying to get the latest trade info, and also the breakeven price.... but the return all show 0.0.
hope that can get help from you, so i can learn from my mistake.